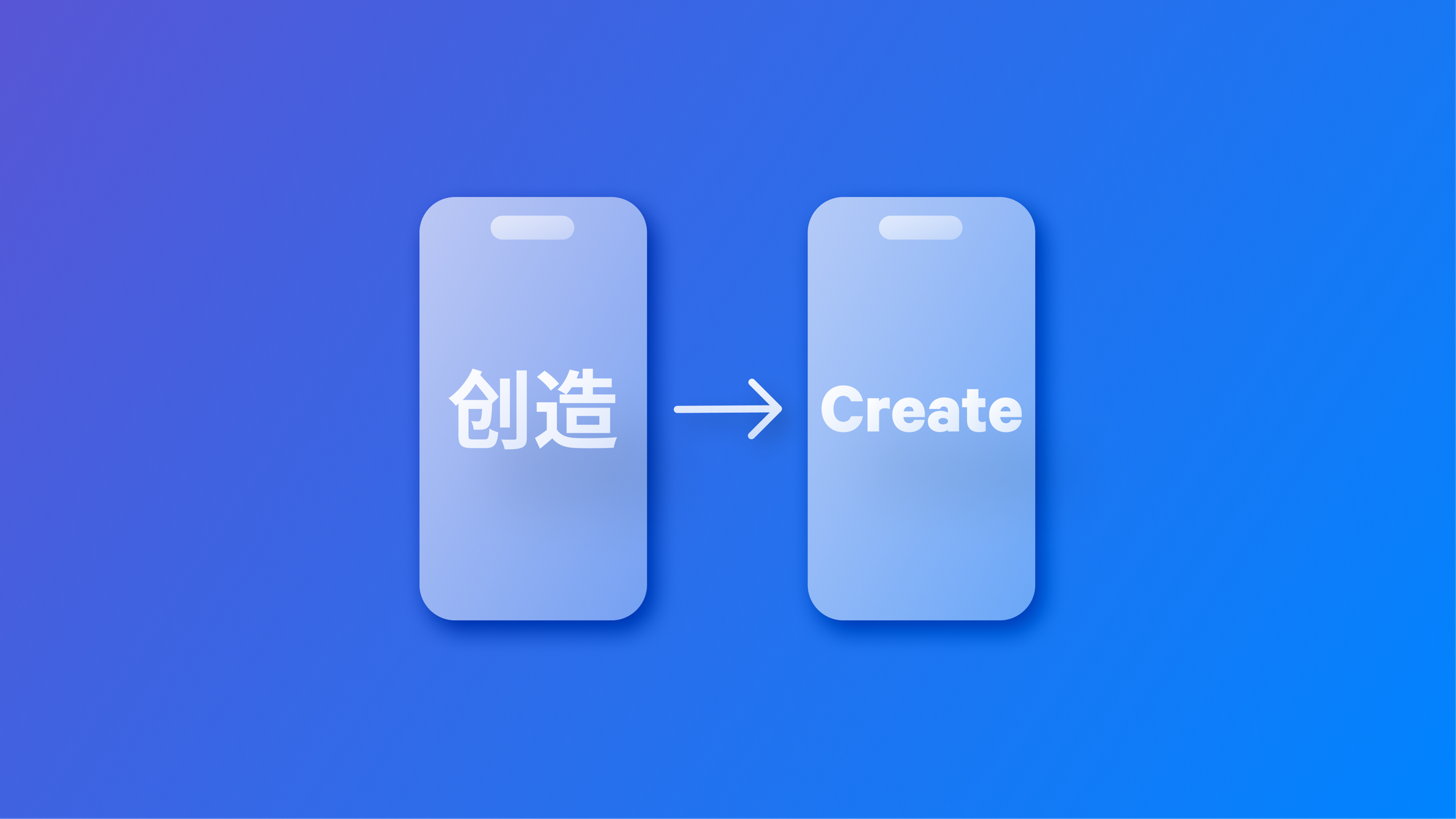
Checking language availability for translation with the Translation framework
Learn how to check availability of a language for translation using the Translation framework.
The new Translation framework uses local machine learning models to translate content inside our apps, but not every language is available for translation. If the user is trying to request a translation of a text from a language that is not yet supported we have to manage it properly.
As a developer, you can address it by using the LanguageAvailability
class from the Translation framework.
Let’s use the LanguageAvailability
class to check which languages are available for translation on the device and if a translation between two different languages is supported.
Create a new SwiftUI View importing the Translation
Framework and create a new instance of the LanguageAvailability()
class.
Print all the languages supported by the Translation Framework by accessing the supportedLanguages
property.
import SwiftUI
import Translation
struct ContentView: View {
private let languageAvailability = LanguageAvailability()
@State private var supportedLanguages: [Locale.Language] = []
private func description(for language: Locale.Language) -> String {
// Used to display the language name
let englishLocale = Locale(identifier: "en-US")
let languageCode = language.languageCode?.identifier ?? ""
let localizedDescription = englishLocale
.localizedString(forLanguageCode: languageCode)
return localizedDescription ?? "No description"
}
var body: some View {
List {
Section {
ForEach(supportedLanguages, id: \.maximalIdentifier) { language in
HStack {
Text(description(for: language))
Spacer()
Text(language.minimalIdentifier)
}
}
} header: {
Text("Supported Languages")
}
}
.task {
self.supportedLanguages = await languageAvailability.supportedLanguages
}
}
}
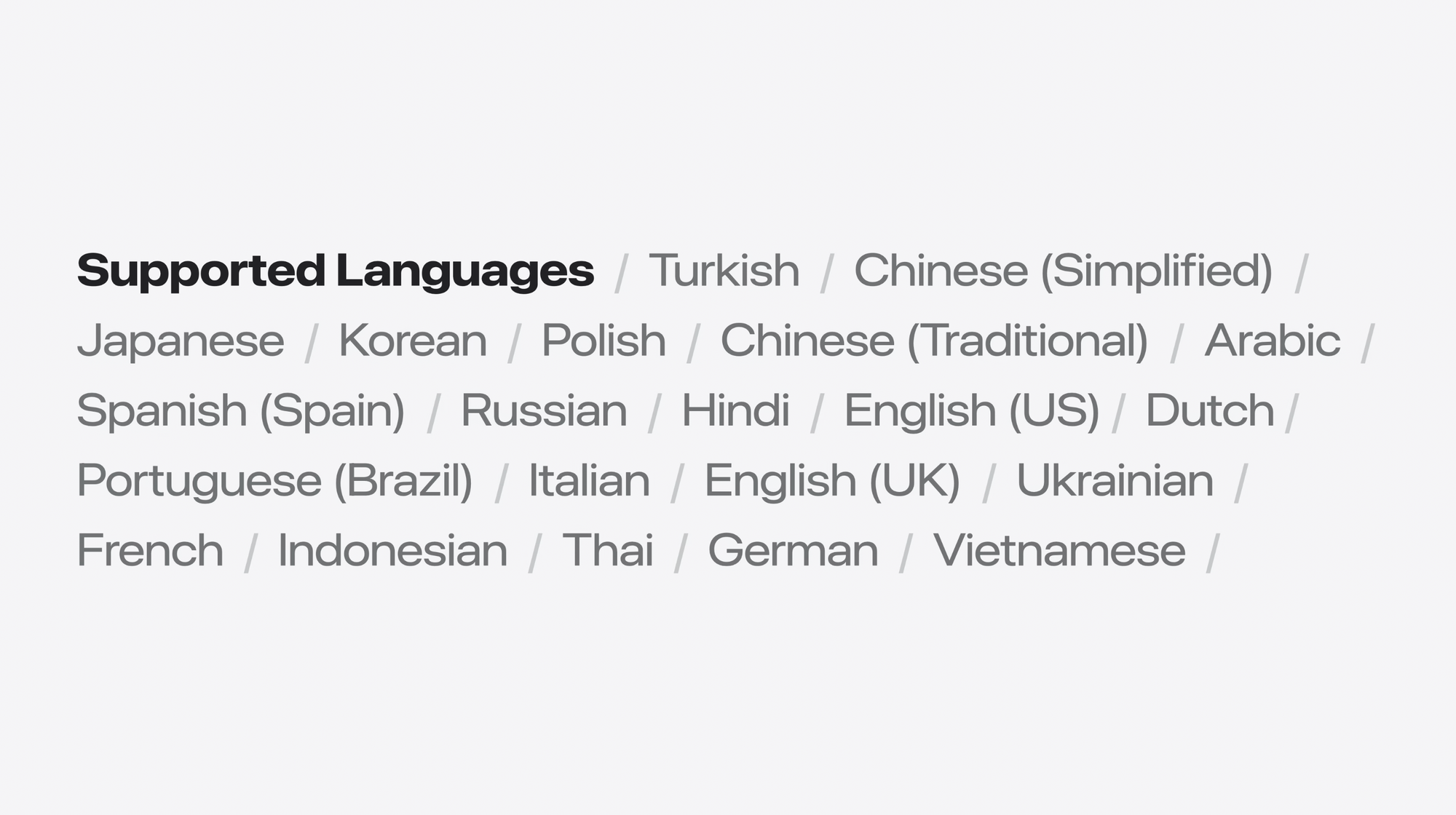
If we want to ensure that a translation from one language to another is supported we can use the status(from:to:)
method. This method will return a LanguageAvailability.Status
value.
private func isTranslationAvailable(
from firstLanguage: Locale.Language,
to secondLanguage: Locale.Language
) async -> Bool {
let status = await languageAvailability
.status(from: firstLanguage, to: secondLanguage)
switch status {
case .installed:
print("Languages installed and translation supported.")
return true
case .supported:
print("Translation supported.")
return true
case .unsupported:
print("Translation unsupported.")
return false
@unknown default:
print("Unknown error.")
return false
}
}
Translation between two languages can be installed
, supported
or unsupported
.
The user can manually remove and download language models from the phone by using the Translate app and accessing the Download Languages option on the navigation bar.
Once a language is downloaded it will be available everywhere across the system.
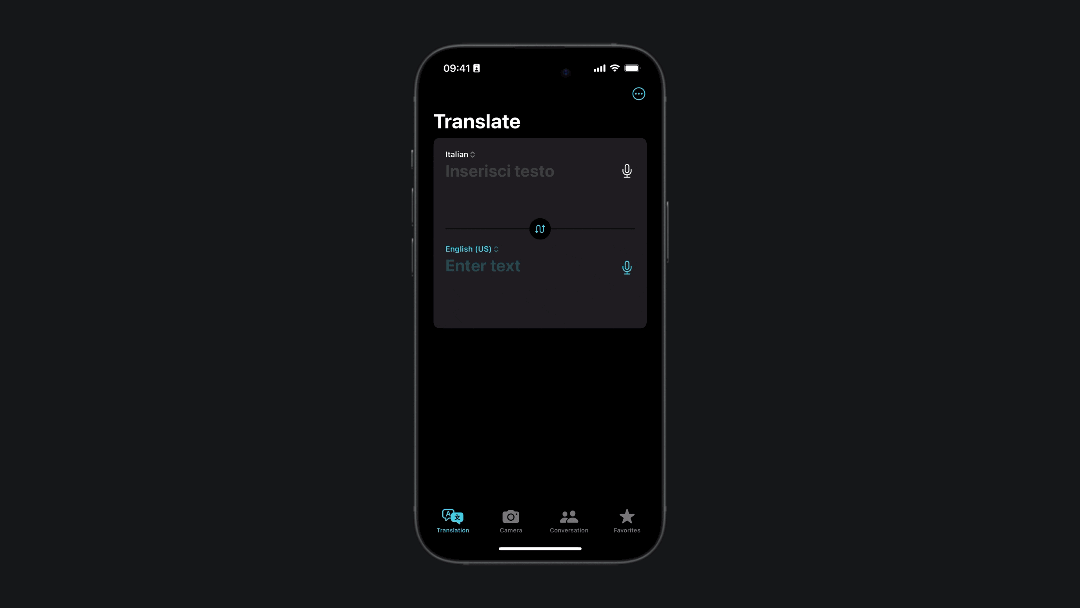