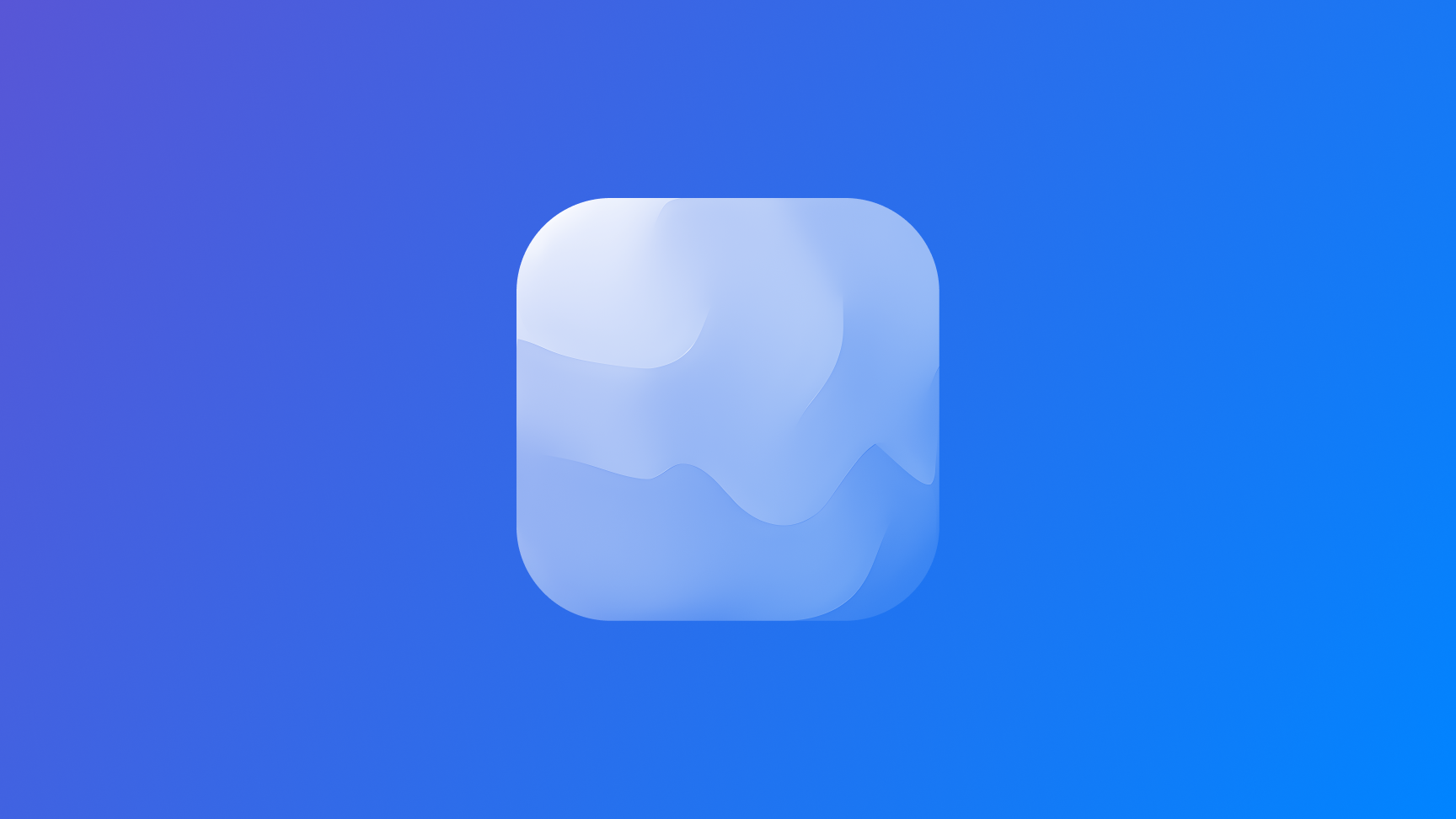
Creating a mesh gradient in SwiftUI
Learn how to create and animate stunning visual effects with Mesh Gradients in a SwiftUI app.
Learn how to create stunning visual effects using SwiftUI's new mesh gradient feature.
Mesh gradients are the most sophisticated type of gradient available in SwiftUI, offering beautiful and complex color transitions rendered with impressive speed. Introduced in iOS 18, mesh gradients provide developers with a powerful tool to create visually striking interfaces and backgrounds.
In this article, we'll explore what mesh gradients are, why they're important, and how to implement them in SwiftUI.
Understanding Mesh Gradients
A mesh gradient is a two-dimensional gradient defined by a 2D grid of positioned colors. Each point in the grid (called a vertex) has a position, a color, and four surrounding Bezier control points that define how it connects to neighboring vertices.
Creating a Simple Mesh Gradient
The easiest way to create a mesh gradient is by specifying the width and height of your gradient, positions for each color, and the colors to display. Here's a simple example:
MeshGradient(width: 2, height: 2, points: [
[0, 0], [1, 0],
[0, 1], [1, 1]
], colors: [.black, .blue, .blue, .black])
In this example:
- We create a 2x2 grid.
- The
points
array specifies the positions of each color usingSIMD2<Float>
values. - The
colors
array specifies the colors for each point.
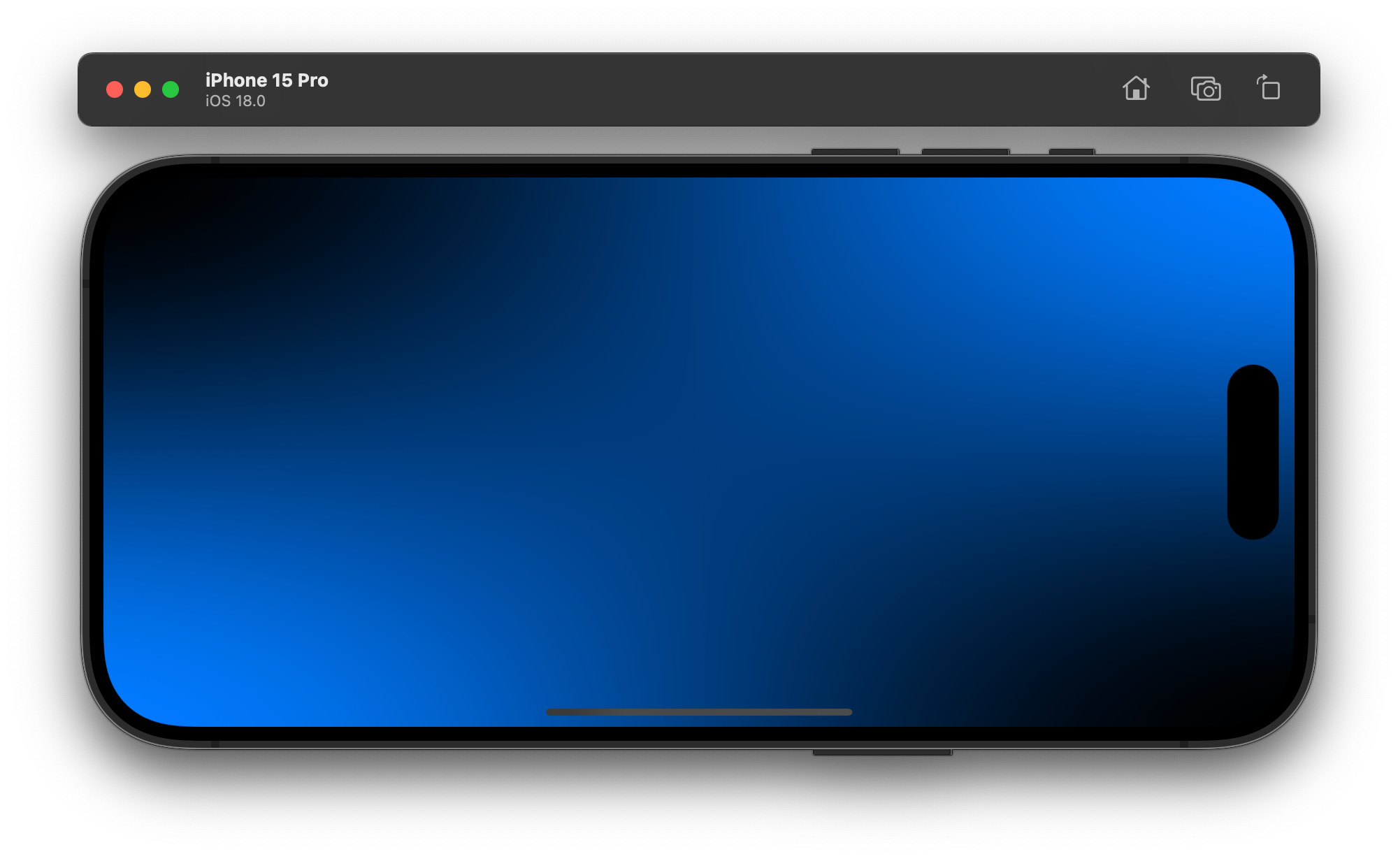
Advanced Mesh Gradient Features
Specifying Background Color
If your points don't cover the full shape of the mesh gradient, you can specify a background color to fill in the missing parts:
MeshGradient(width: 2, height: 2, points: [
[0.1, 0.1], [0.9, 0.1],
[0.2, 0.9], [0.8, 0.9]
], colors: [.black, .blue, .blue, .black], background: .gray)
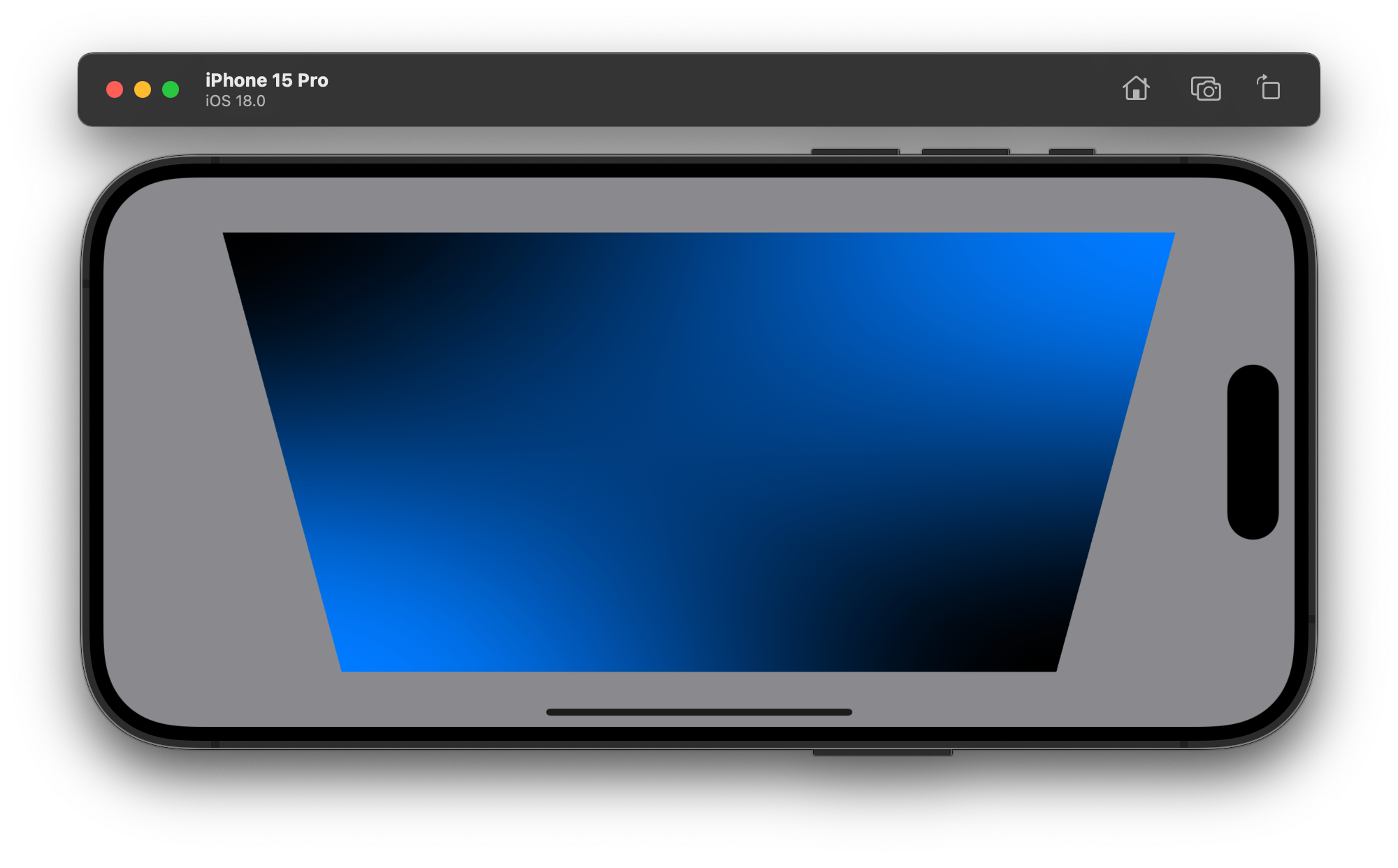
Animating Mesh Gradients
Mesh gradients can be beautifully animated by varying the values you pass in. Here's an example using a TimelineView
:
TimelineView(.animation) { timeline in
let x = (sin(timeline.date.timeIntervalSince1970) + 1) / 2
MeshGradient(width: 3, height: 3, points: [
[0, 0], [0.5, 0], [1, 0],
[0, 0.5], [0.5, Float(x)], [1, 0.5],
[0, 1], [0.5, 1], [1, 1]
], colors: [
.black, .black, .black,
.black, .blue, .black,
.black, .black, .black
])
.ignoresSafeArea()
}
This creates a 3x3 mesh where all elements except the center one are fixed, and the center point moves based on a sine wave.
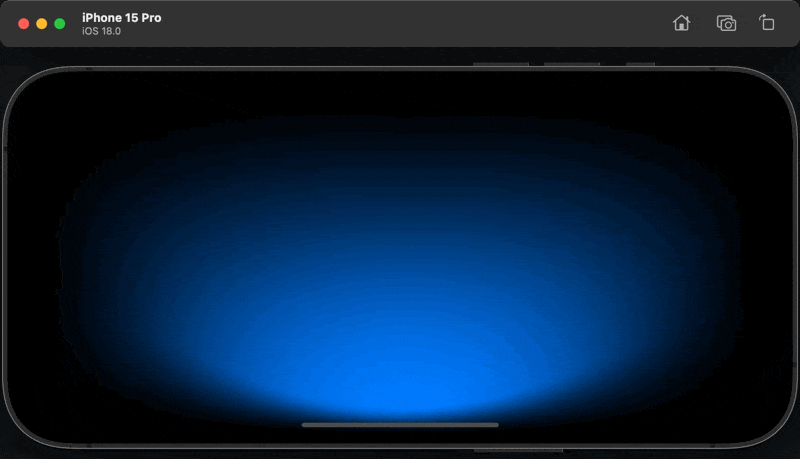
Practical Applications
Mesh gradients are particularly effective as backgrounds and foreground styles for views, including text and icons:
Image(systemName: "sparkles")
.font(.system(size: 144, weight: .black))
.foregroundStyle(
MeshGradient(width: 2, height: 2, points: [
[0, 0], [1, 0],
[0, 1], [1, 1]
], colors: [
.pink, .indigo,
.indigo, .blue
])
)
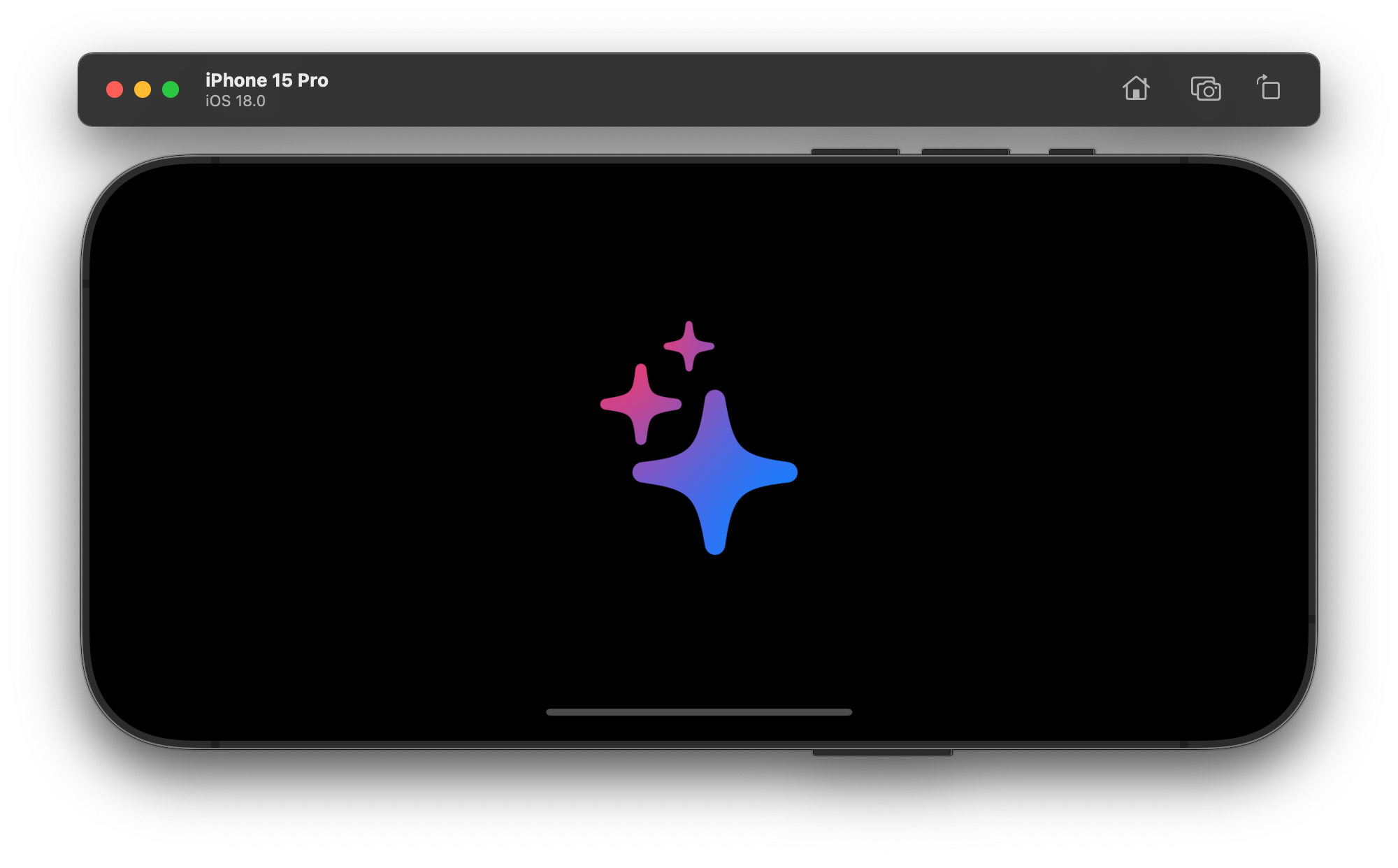
Advanced Options
The MeshGradient
struct offers several advanced options:
smoothsColors
: Determines whether cubic (smooth) interpolation should be used for colors in the mesh.colorSpace
: Specifies the color space in which to interpolate vertex colors.bezierPoints
: Allows explicit specification of Bezier control points for more complex gradients.
Conclusion
Mesh gradients in SwiftUI offer a powerful way to create visually appealing interfaces and effects. By understanding and experimenting with different configurations, you can significantly enhance your app's visual appeal and create unique designs that stand out.
Remember, the key to mastering mesh gradients is experimentation. Try different grid sizes, color combinations, and animations to see what interesting effects you can create!