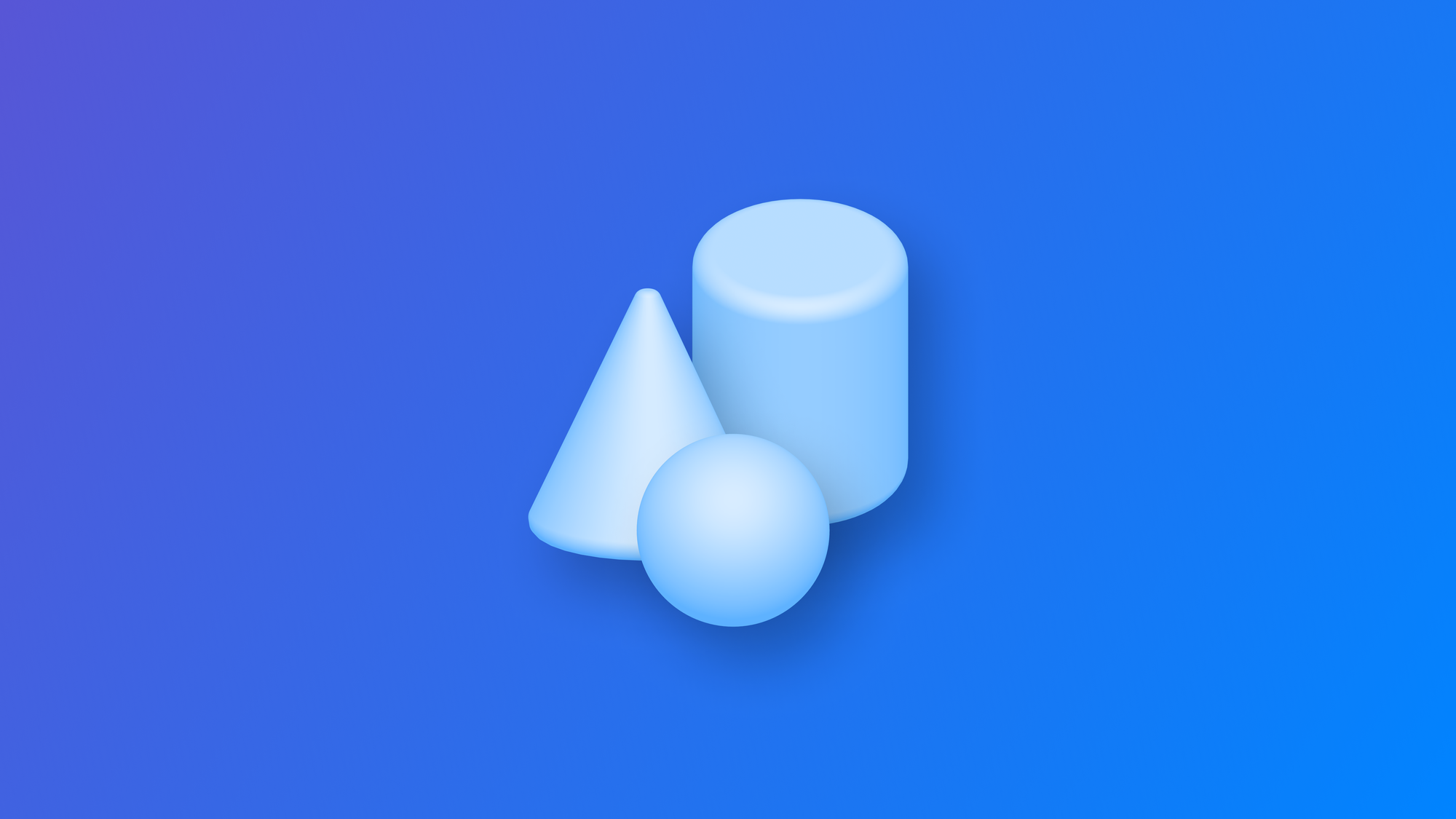
Displaying 3D objects with RealityView on iOS, iPadOS and macOS
Learn how to display 3D models using the RealityKit framework in a SwiftUI app.
During WWDC24 Apple extended the use of RealityView
from VisionOS to the whole Apple Ecosystem (iPhone, iPad, and MacOS). Developers can now create augmented reality apps with just a few lines of code.
Using RealityView
, we can build augmented reality apps using the latest API from RealityKit
without the need of creating UIViewRepresentable
objects or other complex integration steps, allowing developers to focus more on designing engaging AR experiences rather than dealing with the technical details behind it.
In this reference, we show how to use RealityKit to create a simple augmented reality app. For this sample project, we will require a 3D model to be showcased in augmented reality. You can find a variety of downloadable models on the Apple Quicklook website.
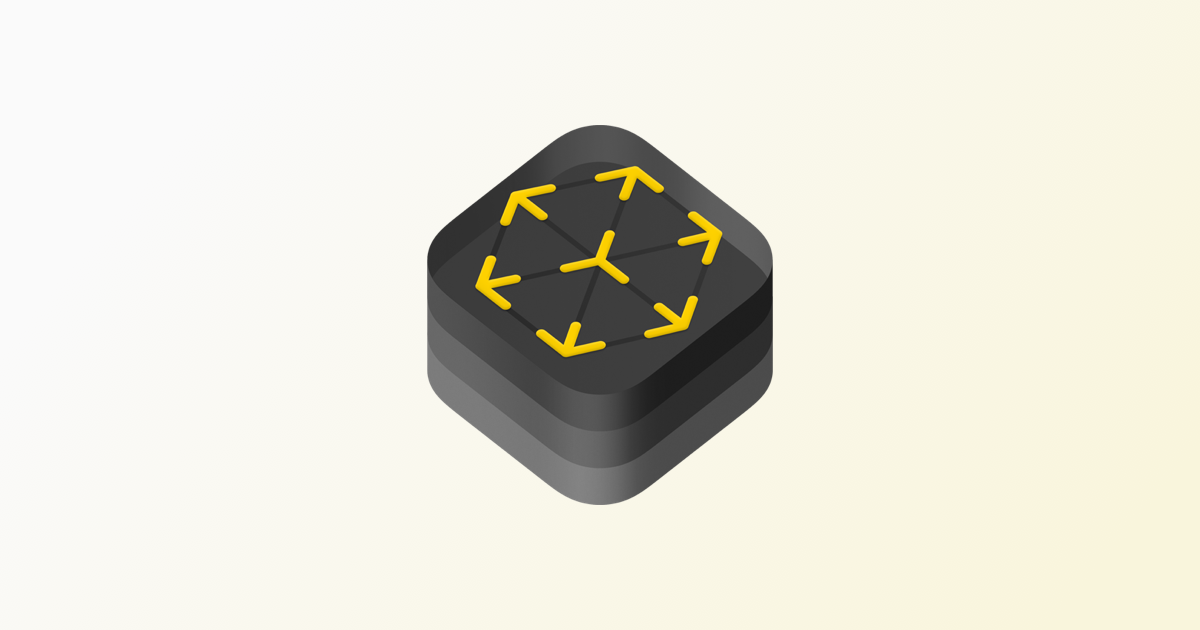
Privacy Options
Before diving into the code make sure also to enable the camera access by going into the Info.plist file of your project. You must ensure that users are informed and have control over how their device's camera is used.
- Go into your project settings and navigate to the Info tab, as part of your project’s target;
- Add a new Key in the Custom iOS Target Properties:
Privacy - Camera Usage Description
; - Add a string value describing why the app needs access to the camera.
Implementation
import SwiftUI
import RealityKit
struct RealityComponent: View {
var body: some View {
VStack {
RealityView { content in
content.camera = .worldTracking
if let airplane = try? await ModelEntity(named: "airplane") {
content.add(airplane)
}
}
}
}
}
The RealityView
container is used to display all our RealityKit
entities. In the asynchronous closure, the RealityView
builds the starting content. On iOS, iPadOS, and macOS the content of the RealityView
is of type RealityViewCameraContent
.
By setting the camera
property as worldTracking
the system will automatically access the camera and start analyzing the environment. After that, add the model to the content of our RealityView
using the add(_:)
method.
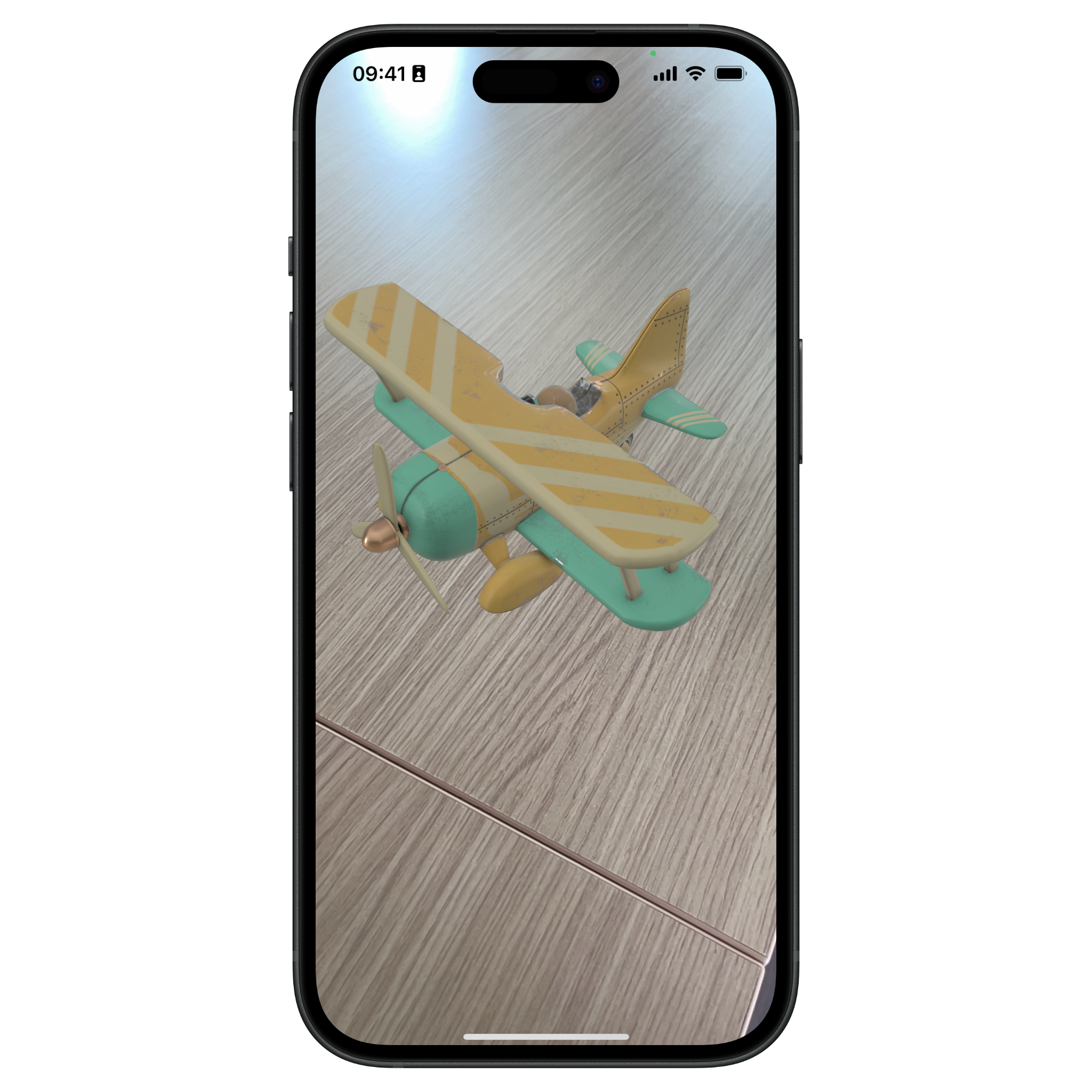
It is also possible to display the 3D model in a non-AR mode by setting the camera
property as virtual
. It means that RealityKit will render the 3D model directly inside the View.
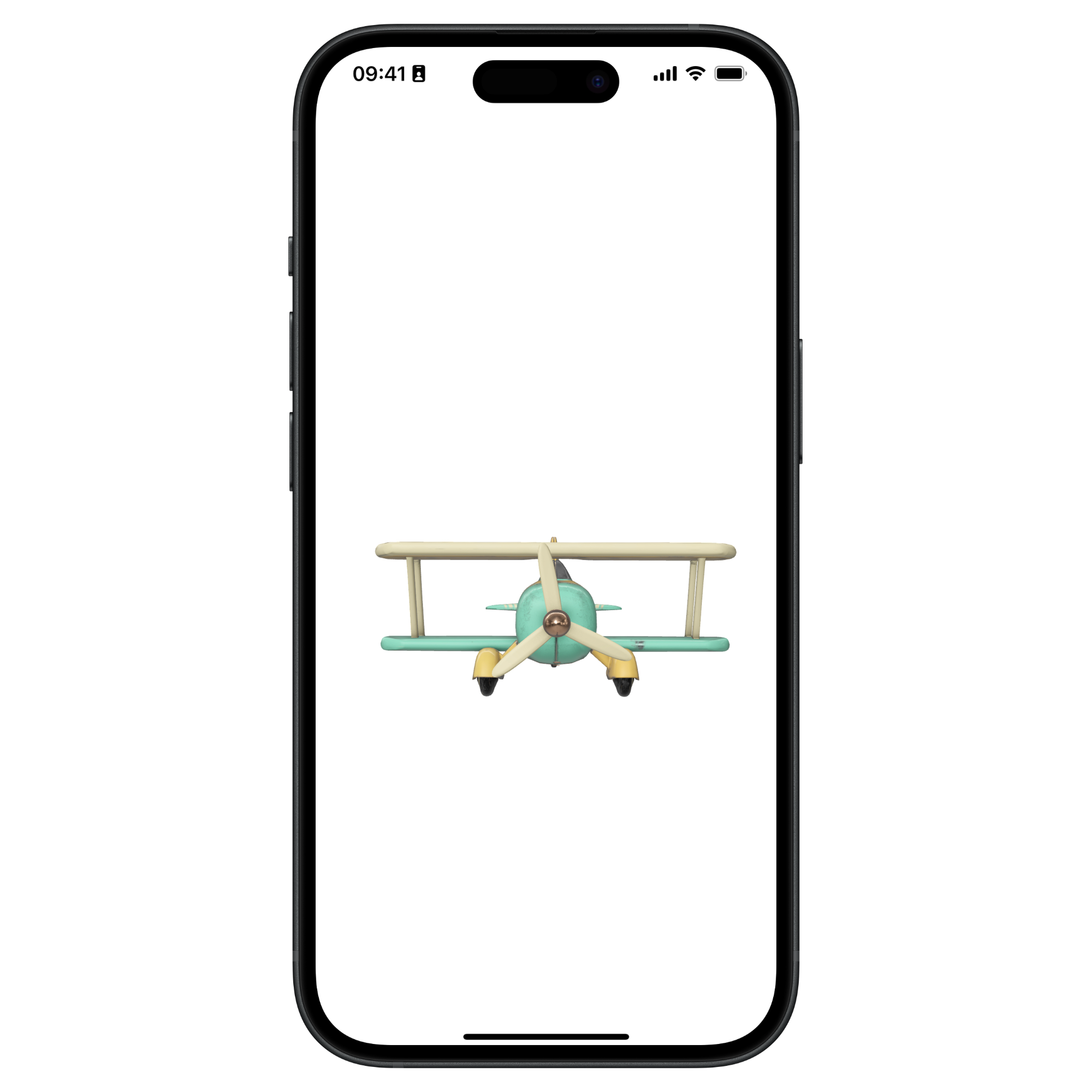
Using the RealityView component, we can build augmented reality apps using the latest API from RealityKit
without the need to create UIViewRepresentable
objects or other complex integration steps allowing developers to focus more on designing engaging AR experiences rather than dealing with technical details.