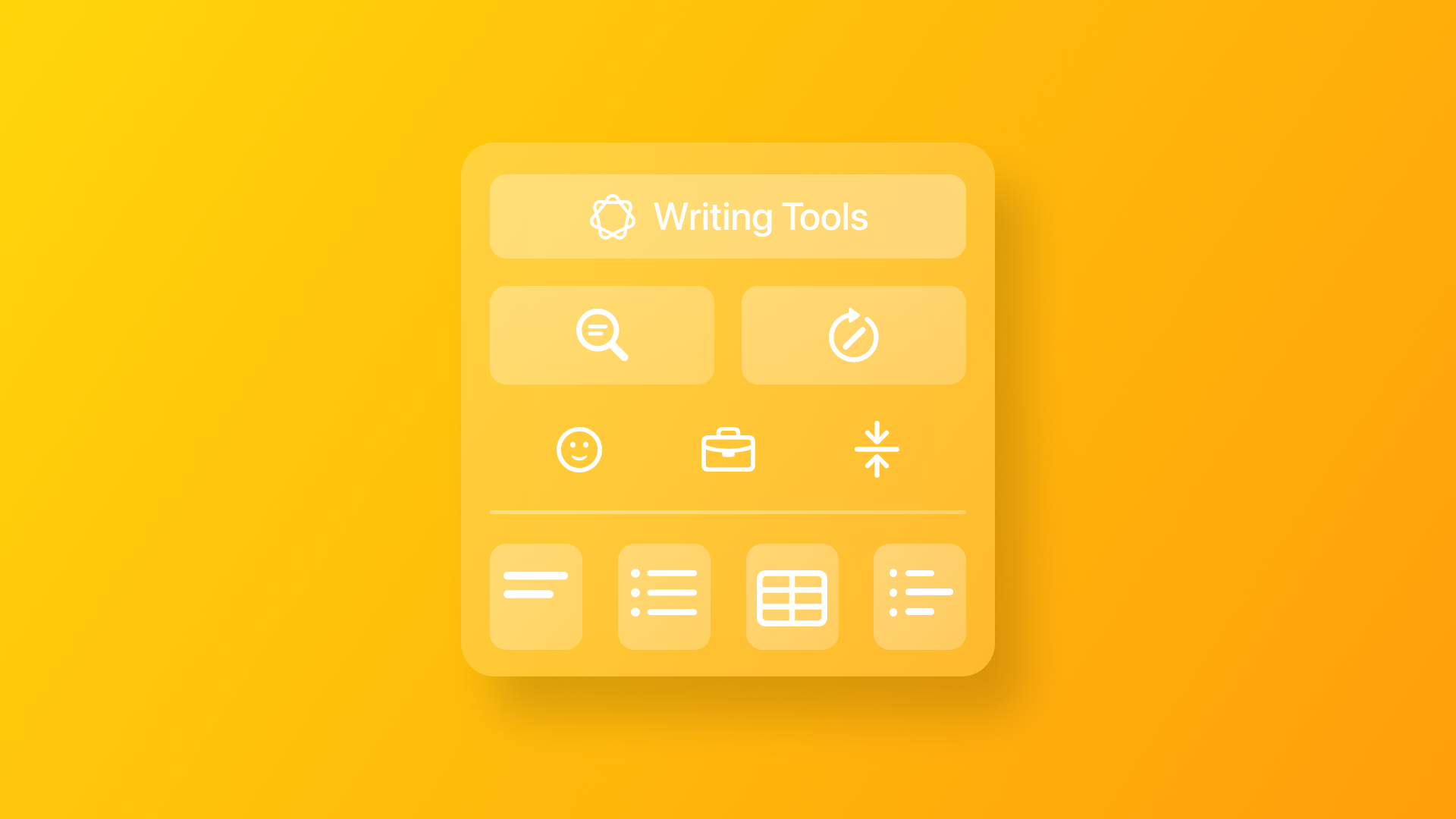
Exploring Apple Intelligence: Writing Tools
Understand Writing Tools, powered by Apple Intelligence.
The introduction of Apple Intelligence enhances the writing experience with its innovative features. Writing Tools is an advanced AI-powered toolset to empower users to write more easily, accurately, and efficiently across Apple devices. They assist users in composing, editing, and refining their writing seamlessly, whether they’re working on emails, documents, messages, or notes.
In this article, we’ll delve into Writing Tools, explore its integration, and provide insights for developers on how to leverage it in their applications.
The Writing Tools
When users select a text block the Apple Intelligence icon will be displayed. On iOS it will appear at the callout bar or the toolbar. On macOS it can appear in the context menu and the edit menu. This icon provides easy access to a comprehensive set of features.
This functionality extends to non-editable text as well, where results of the writing operations appear in a panel where users can share or copy the text. This ensures that users can still benefit from the intelligence enhancements, regardless of the text being editable or not.
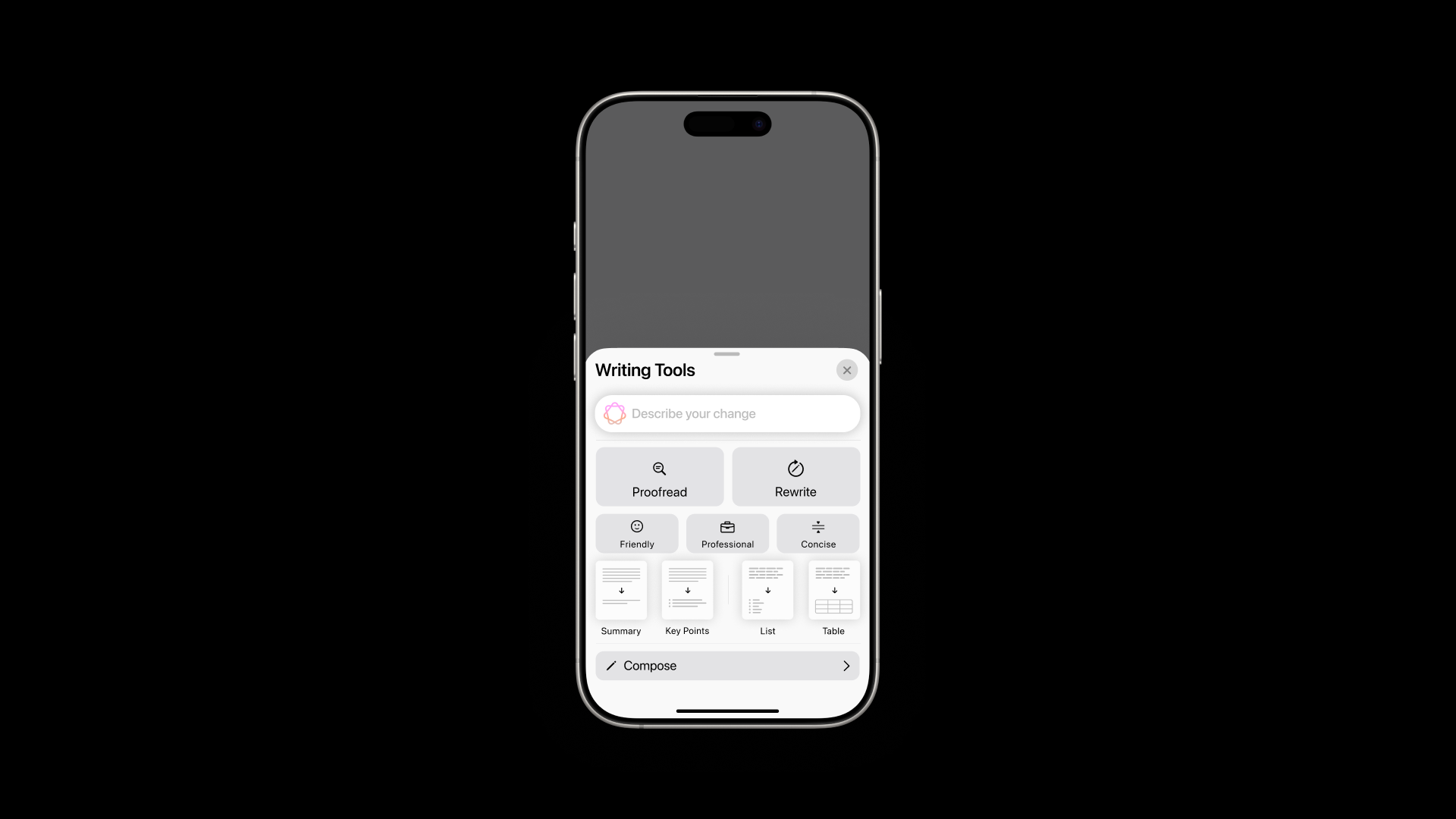
Let’s see what these features are and what they allow the user to achieve.
Proofreading
It identifies and corrects grammatical and spelling errors, highlighting the corrections with a smooth, animated underline for easy comprehension. Users can review the changes by tapping on each one or directly browsing the sheet that slides up from the bottom of the screen.
For each change, whether it’s a misused phrase, misspelling, or missing punctuation, Apple Intelligence offers an explanation and a quick option to revert to the original version. Additionally, the panel’s navigation bar allows users to accept or reject all changes simultaneously, streamlining the proofreading process and ensuring precision.
Rewriting
It simply rephrases the text. When a single word is selected, the tool intuitively extends the selection to encompass a larger text chunk, ensuring contextually appropriate suggestions.
It is possible to adjust the text's tone while rewriting choosing among friendly for a more casual style, professional for a more formal approach, and concise to shorten the text.
Text Transformation
It enables the automatic transformation of text into key points, lists, or tables, ensuring a clear and structured layout.
Summarizing
It helps users condense long text into concise summaries, enhancing readability and quick understanding.
Compose with ChatGPT
Enabling ChatGPT on your device allows you to receive assistance with text composition, enabling more personalized content creation. Users can prompt ChatGPT to generate text in various styles, tones, or formats, facilitating the rapid creation of drafts and creative content.
Prompting AI
This feature will enable users to specify the desired type of modification directly, providing greater precision over the final output.
Enhancing UX with Intelligent Animation
Animations play a key role in signaling to users that Apple Intelligence is actively working behind the scenes within the Writing Tools. From the moment a session begins, subtle animations indicate that processing is underway, giving users clear visual feedback that Apple Intelligence model is engaged and assisting. For example, during text proofreading and rewriting, animated highlights appear on the selected text, underscoring that the tool is actively assessing and refining content.
When changes are made, such as in proofreading, these modifications are displayed with a smooth, animated underline, making it evident where Apple Intelligence has detected and corrected potential issues. By delivering rewritten text in segments, especially for longer passages, the animation helps prevent abrupt changes, making the AI’s involvement seamless and reassuring.
Overall, these carefully designed animations contribute to a transparent and user-friendly experience, allowing users to intuitively sense when Apple Intelligence is enhancing their writing in real time.
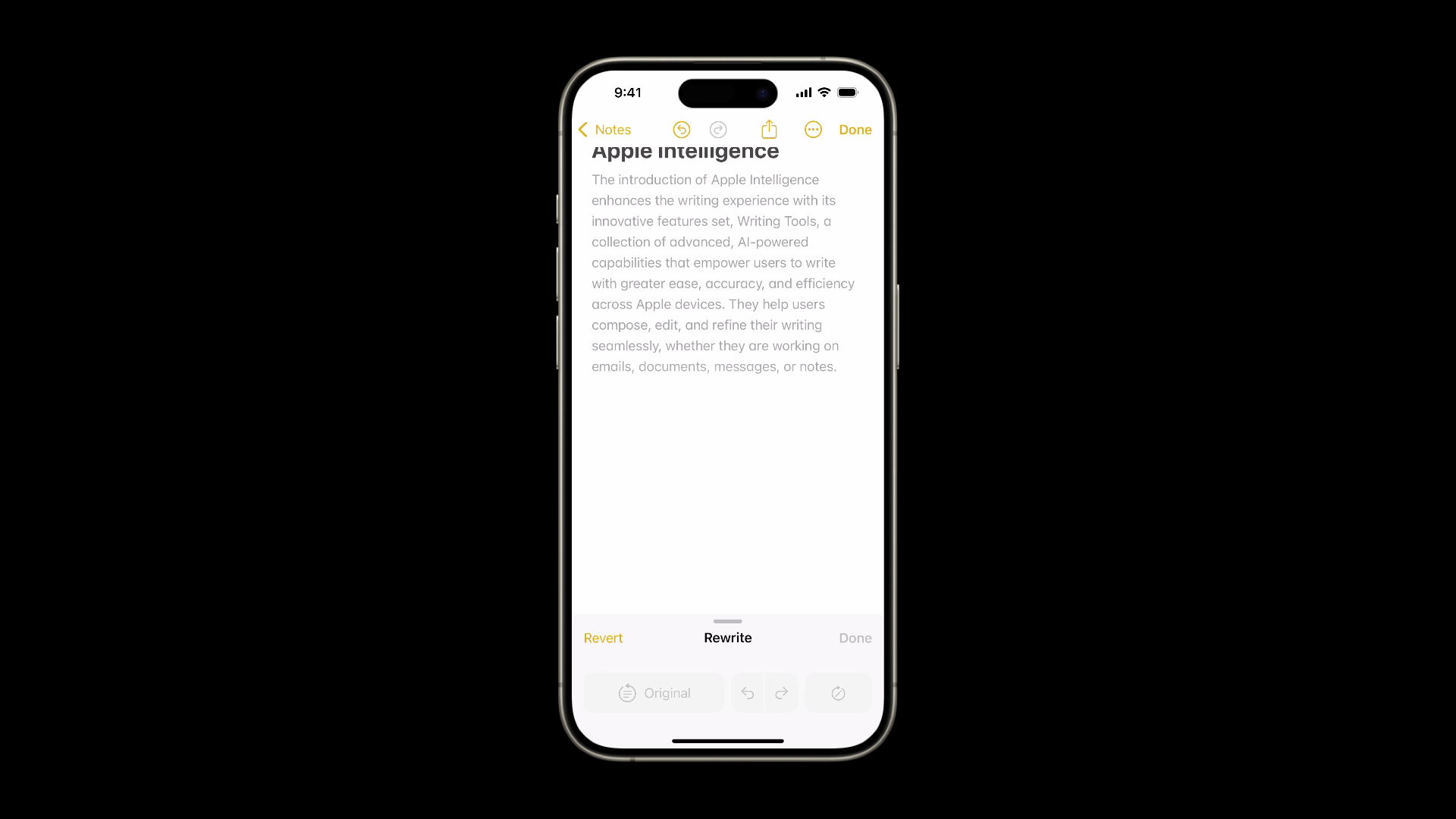
Seamless Integration Across Apple’s Ecosystem
Apple Intelligence’s Writing Tools integrate smoothly across Mail, Messages, Notes, and Safari, enhancing the user experience by providing powerful support for refining and summarizing content in each app.
In Mail, Writing Tools assist in refining email drafts, a summary feature generates a brief overview beneath each email in the inbox, providing a quick snapshot of the content and users can generate full summaries of email threads.
In Messages, it allows to refine message drafts, and a summary feature displays a concise recap of unread messages, helping users stay on top of conversations with ease - this feature also applies to notifications in general.
In Notes, users can use the full suite of features, providing them extensive editing capabilities with the summary of the transcribed content recorded directly by using the audio recording feature integrated into the app.
In Safari, Writing Tools empower users to summarize the content of the webpage content.
The Writing Tool capabilities are automatically integrated into UI native components such as Text
, TextField
, and TextEditor
. As a built-in system feature, Writing Tools ensures developers don’t need to update their apps to maintain compatibility. However, certain APIs enable customization, allowing us to adjust the Writing Tools’ behavior specifically within our app.
Writing Tools within SwiftUI
As said before the Text
, TextField
and TextEditor
views already fully support Writing Tools. As developers, one thing that can be customized is the type of experience that we want to provide. We can do that by using the writingToolsBehavior(_:)
modifier passing a WritingToolsBehavior
value.
There are four behavior options:
automatic
: the type of editing assist will be automatically decided by the systemcomplete
: enables full editing capabilities with automatic text replacementdisabled
: disables all editing assistance tools for a specificView
limited
: offers a simplified, overlay-based editing experience where possible, providing some basic styling options while restricting more advanced tools.
import SwiftUI
struct ContentView: View {
@State var message: String
var body: some View {
VStack {
TextEditor(text: $message)
/// Defining the writing tools behaviour for the text
.writingToolsBehavior(.complete)
/// Aesthetic modifiers
.cornerRadius(8)
.frame(height: 300)
.padding(.horizontal)
.border(.blue)
}
.padding()
}
}
Writing Tools within UIKit
UIKit provides greater flexibility in controlling various stages of the Writing Tools process, allowing for more interactions and adjustments throughout each phase.
import SwiftUI
import UIKit
struct CustomTextView: UIViewRepresentable {
@Binding var text: String
func makeUIView(context: Context) -> UITextView {
let textView = UITextView()
textView.text = text
textView.isEditable = true
textView.font = UIFont.systemFont(ofSize: 17, weight: .regular)
textView.delegate = context.coordinator
return textView
}
func updateUIView(_ textView: UITextView, context: Context) {
textView.text = text
if textView.isWritingToolsActive {
print("Writing Tool is active")
}
}
func makeCoordinator() -> Coordinator {
Coordinator()
}
class Coordinator: NSObject, UITextViewDelegate {
var writingToolsResultedText = ""
func textViewWritingToolsWillBegin(_ textView: UITextView) {
writingToolsResultedText = textView.text
print("Writing Tools will begin")
}
func textViewWritingToolsDidEnd(_ textView: UITextView) {
if textView.text != writingToolsResultedText {
print("Text changed with Writing Tools")
}
}
}
}
In the example above by using a coordinator conforming with the UITextViewDelegate
protocol we can access two specific phases of the Writing Tool process.
When the editing process of the Writing Tools starts the textViewWritingToolsWillBegin(_:)
method will be invoked allowing to perform specific actions in this stage. The textViewWritingToolsDidEnd(_:)
method will be invoked when the editing process is finished.
Additionally, we can also access to the isWritingToolsActive
property to understand if the writing tools are currently interacting with the text view’s content.
Setting Writing Tools behavior
As we saw in the writingToolsBehavior(_:)
modifier available for SwiftUI we can do the same with the UITextView
object in UIKit by setting the writingToolsBehavior
property to a specific value.
import SwiftUI
import UIKit
struct CustomTextView: UIViewRepresentable {
@Binding var text: String
func makeUIView(context: Context) -> UITextView {
...
textView.writingToolsBehavior = .complete
return textView
}
...
}
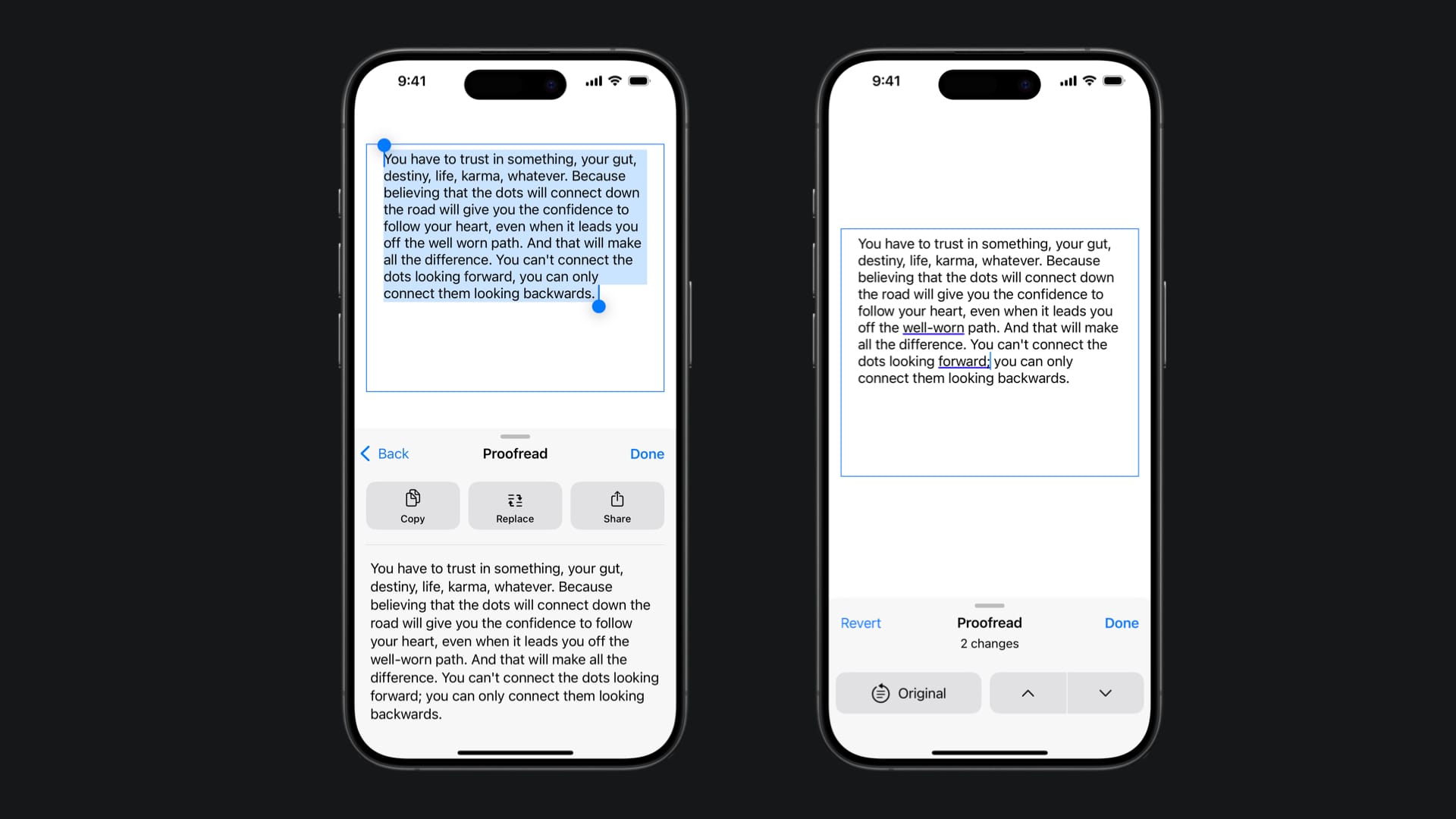
Result option
You can also filter the editing output of Writing Tools on a UITextView
by assigning an array of UIWritingToolsResultOptions
objects. This configuration allows you to specify which types of editing outputs are available, providing more control over the text modifications that users can apply within the text view.
import SwiftUI
import UIKit
struct CustomTextView: UIViewRepresentable {
@Binding var text: String
func makeUIView(context: Context) -> UITextView {
...
textView.allowsEditingTextAttributes = true
textView.allowedWritingToolsResultOptions = [
.plainText,
.list,
.richText
]
return textView
}
func updateUIView(_ textView: UITextView, context: Context) { ... }
func makeCoordinator() -> Coordinator { ... }
class Coordinator: NSObject, UITextViewDelegate { ... }
}
When you use this option is always recommended to set allowsEditingTextAttributes
to true so that the Writing Tools are allowed to edit text attributes (such as font, color, or style) directly within the text view.
Selectable and not selectable text
When text is not selectable Writing Tools will not automatically replace the textual content but a popup will be presented with the possibility of copying the result. The following example has the property isEditing
of the textView
set to false.
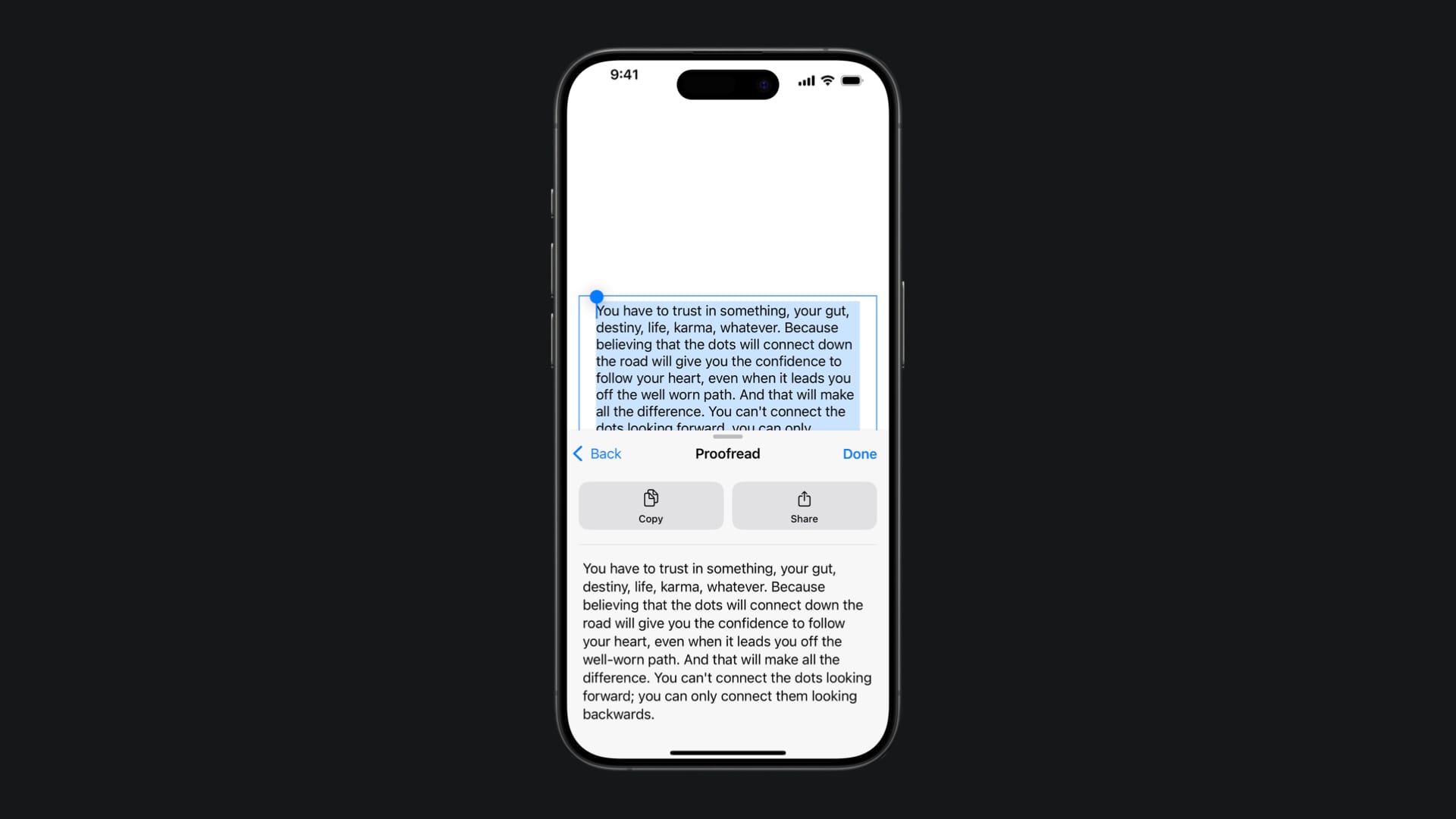
Writing Tools provides a new suite of features aimed at making working with text a better experience for users. From a development point of view, they are still limited in ensuring that the areas of the app where they are relevant support them well.
In the future, developers would benefit from access to APIs that allow a further level of customization or even using Writing Tools to provide summarization and transformation tasks without the need for a user prompt.