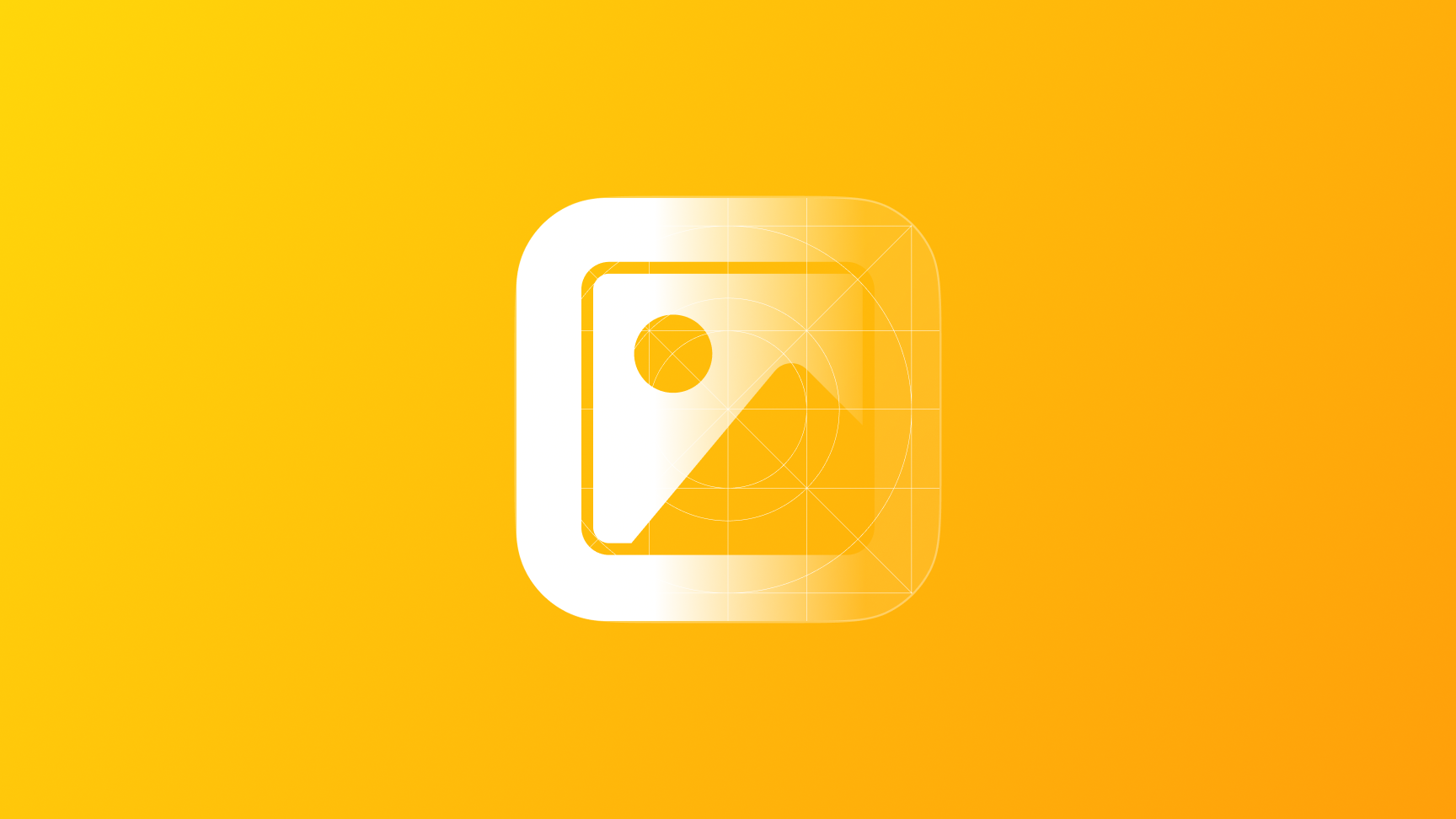
Getting started with Core Image
Take your first steps into the world of image processing within the Apple ecosystem with the Core Image framework.
Core Image is a powerful image processing and analysis framework developed by Apple for its platforms. It provides developers with high-performance tools for processing still and video images, applying filters, and performing complex image manipulations.
In this guide, we'll explore the basics of Core Image, the evolution of the framework, and how to get started with it.
What is Core Image?
Introduced in 2005 with macOS 10.4 Tiger, Core Image is an image processing and analysis framework that stands out for its high performance. Originally part of the QuartzCore framework – now referred to as Core Animation – it enabled near-real-time non-destructive image processing, and seamlessly filters and effects applications within the Quartz graphics rendering layer – now known as Core Graphics.
In 2011, these image processing capabilities were brought to mobile devices with the integration of the framework in iOS 5. Like Core Audio, the framework's architecture was designed to support a plugin-based system for applying filters, which allowed for flexibility and extensibility in how images could be processed.
Advancements in technology led Apple to the introduction of the Metal framework for advanced 3D graphics rendering and parallel data computation with graphics processors, which Core Image now leverages to improve performance.
Core Image initially played a role also in computer vision tasks like face detection. However, the introduction of the Vision framework and Core ML for more advanced computer vision tasks shifted its primary focus to applying visual effects and transformations.
Today, Core Image remains essential for image manipulation, allowing real-time effects and enhancements through high-performance processing and fast image rendering also thanks to its leverage of the GPU. Also, its wide collection of built-in image filters, ready to be applied to images and chained together, makes room not only for the creation of complex effects but also for filter customization by using the Core Image Kernel language or Metal, for specific needs.
Last but not least, its integration with other Apple frameworks like AVFoundation and UIKit, makes it easy to be implemented into applications that require image or video processing.
Main Components of Core Image
To use Core Image effectively, it’s essential to understand its fundamental building blocks. These components work together seamlessly to process and render images efficiently.
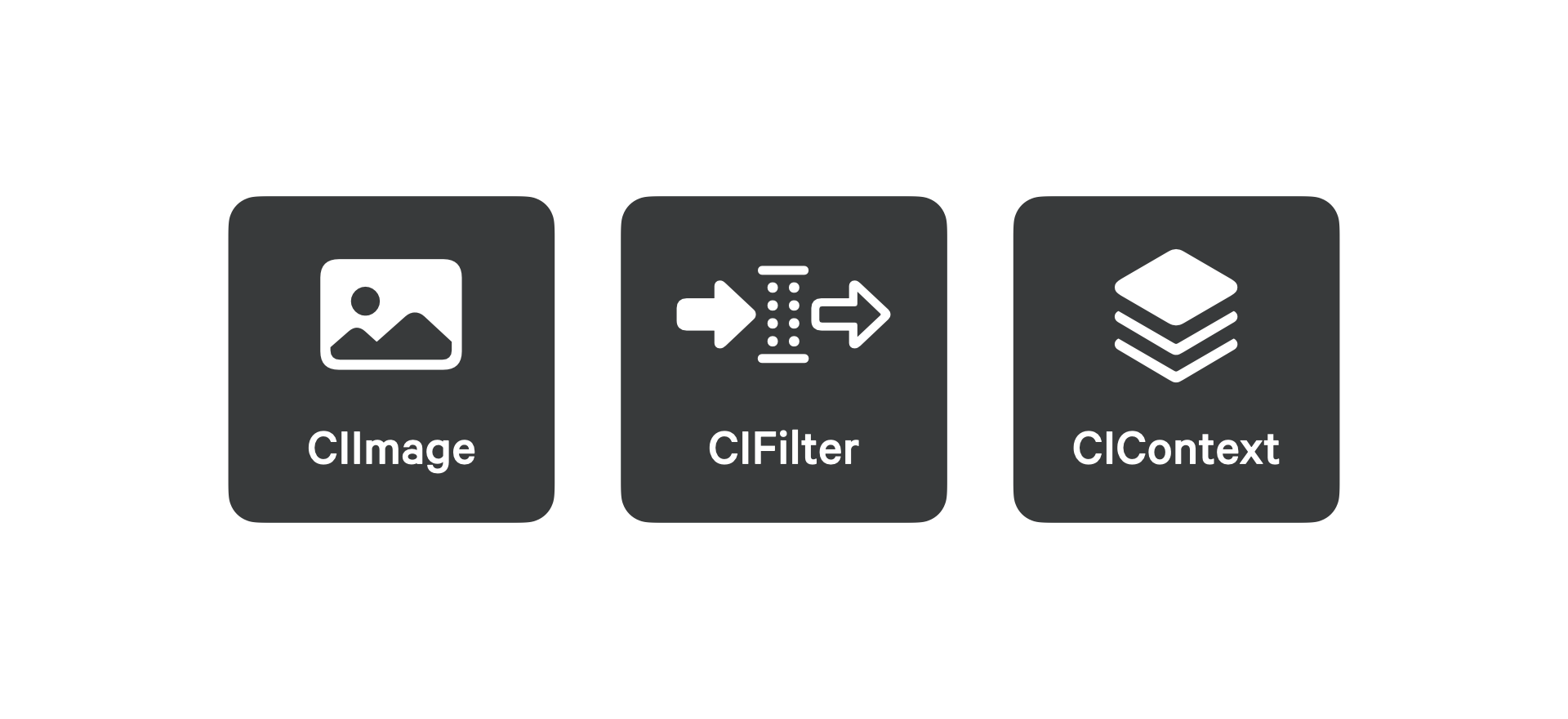
Core Image's architecture is built around three main components:
CIImage
: Represents the image data used for processingCIFilter
: Applies effects or transformations to imagesCIContext
: Manages the rendering of processed images
CIImage
The class CIImage
is fundamental in Apple’s Core Image framework, representing the image data used for processing. Contrary to what the name might suggest, a CIImage
is not a traditional image that stores pixel data directly like it happens with CGImage
from Core Graphics. Instead, it serves as an immutable set of data and instructions that describes how to produce an image when required. The actual rendering occurs only when explicitly requested, enabling lazy evaluation that optimizes performance and resource usage while maintaining the integrity of the original image data after the processing.
A CIImage
can be created from different sources such as from existing images (like UIImage
and CGImage
), directly from image files and from raw pixel data.
import CoreImage
// From UIImage
if let inputUIImage = UIImage(named: "image-example"),
let ciImage = CIImage(image: inputUIImage) {
print("Successfully created CIImage from UIImage")
}
// From a file URL
if let imageURL = Bundle.main.url(forResource: "image-example", withExtension: "jpg"),
let ciImage = CIImage(contentsOf: imageURL) {
print("Successfully created CIImage from file URL")
}
// From image data (e.g., from a network response)
let imageData: Data?
if let imageData = imageData,
let ciImage = CIImage(data: imageData) {
print("Successfully created CIImage from image data")
}
CIFilter
A CIFilter
, as the name suggests, is a filter that can be applied to a CIImage
. It takes one or more input images, processes them by applying specific transformations, and produces another CIImage
as its output.
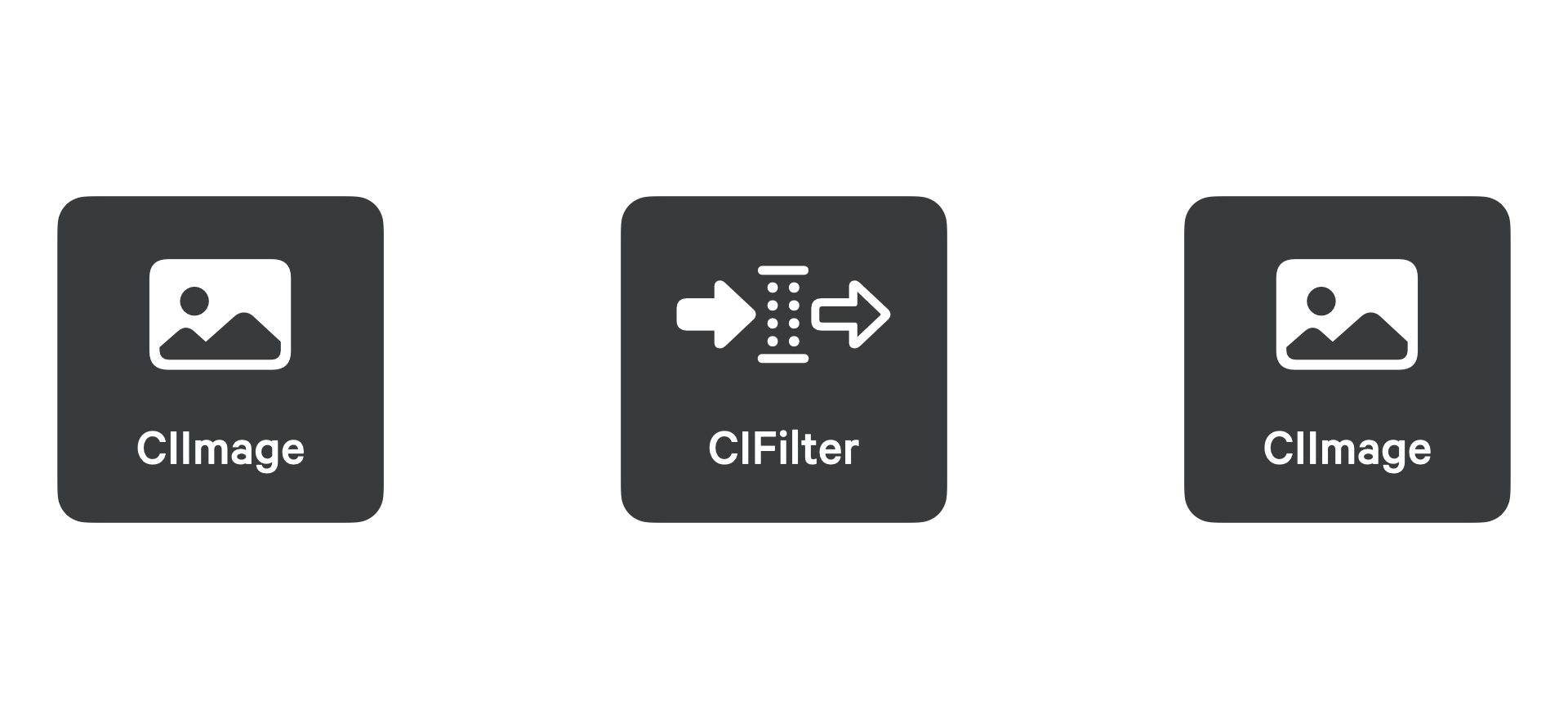
This means it doesn’t immediately generate a rendered image but instead updates the instructions for how the image should be processed. This approach allows for efficient and non-destructive processing, as filters modify the processing instructions rather than altering the original image data directly.
As mentioned earlier, Core Image’s architecture is designed to support a plugin-based system for applying filters, making it both flexible and extensible. Developers can chain multiple filters together to create complex effects, with each filter adding a new step to the image processing workflow.
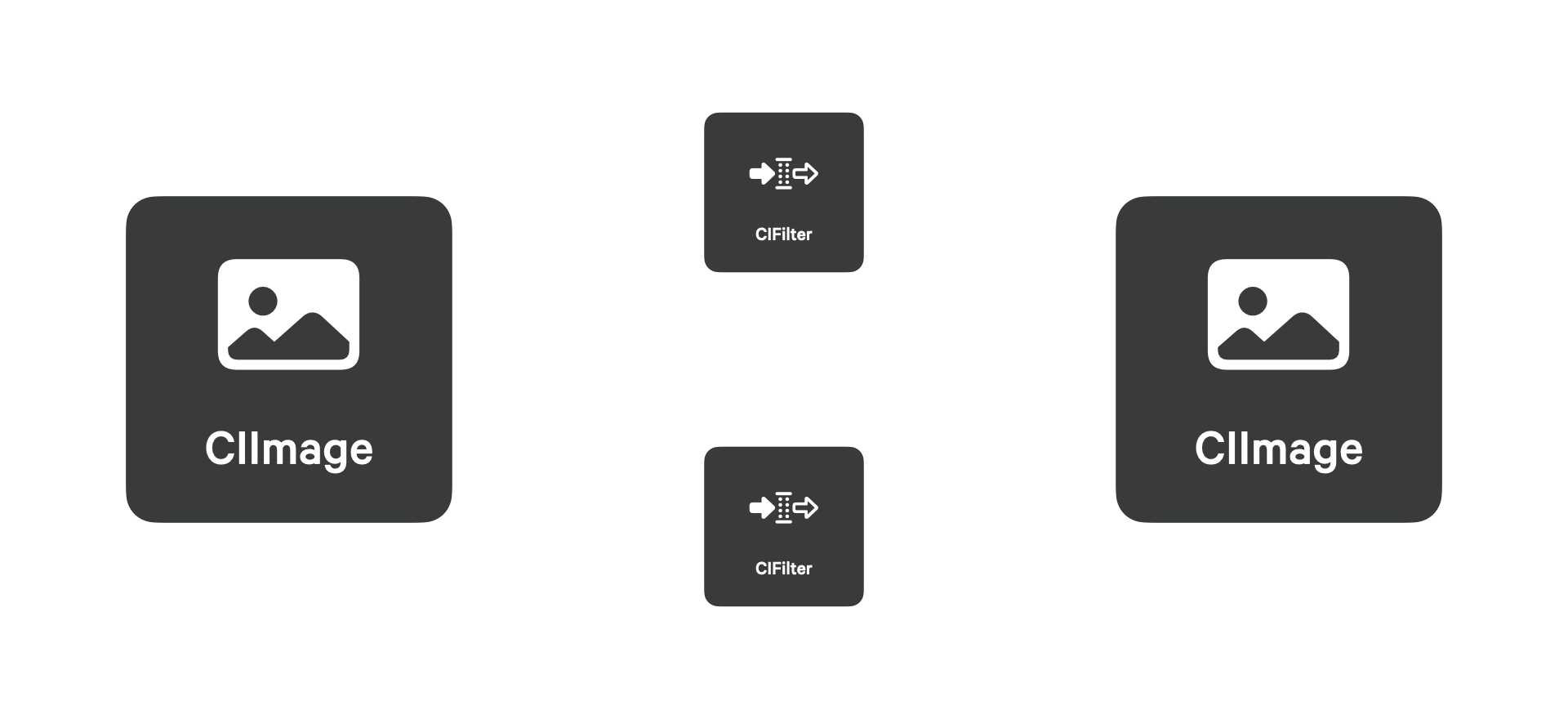
Core Image provides a comprehensive set of built-in filters, categorized by their effects, such as color adjustments, distortions, blurs, and more.
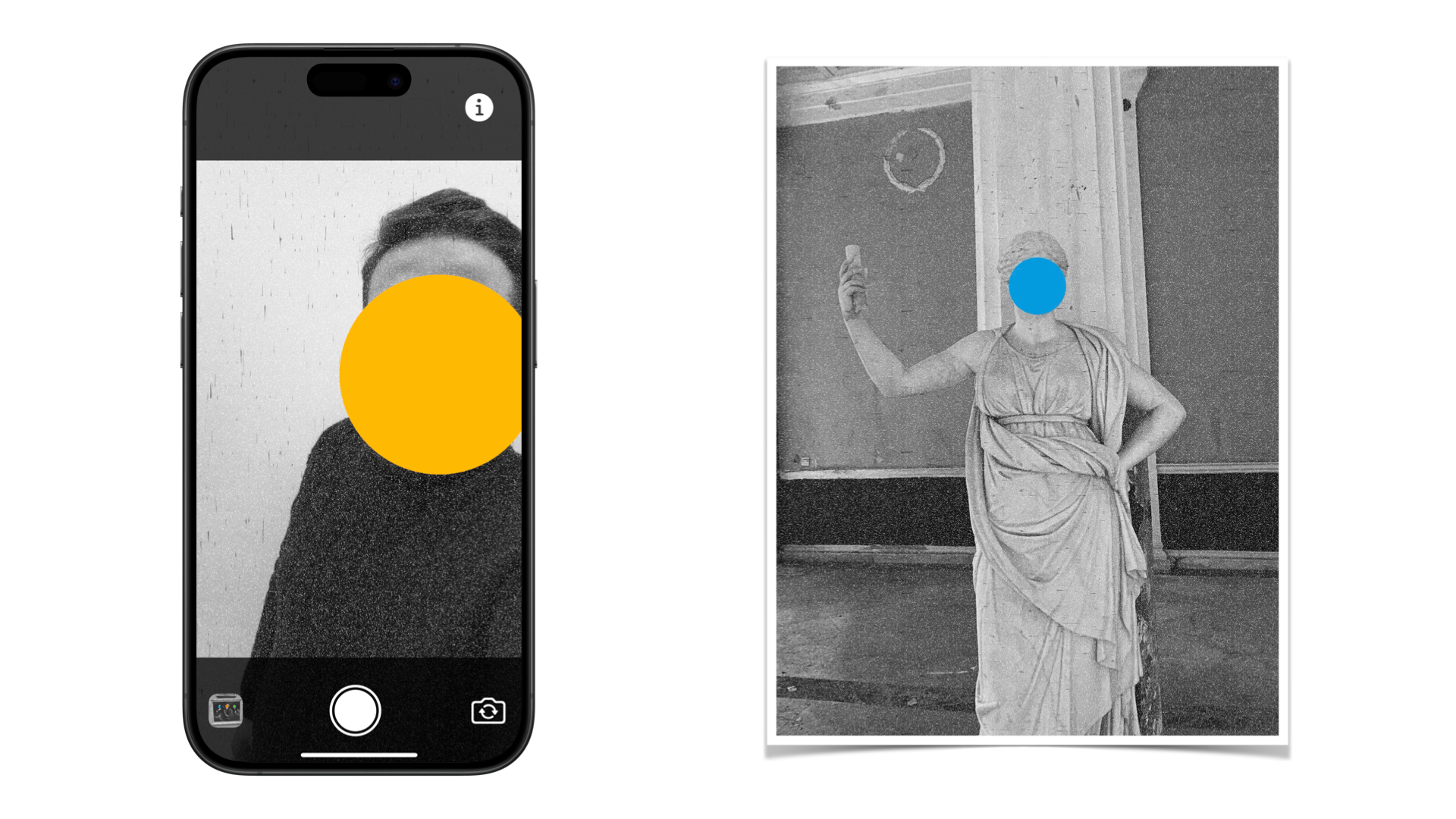
For added flexibility, developers can create custom CIFilter
s by combining built-in filters or writing custom kernels. This extends the framework’s capabilities, enabling tailored image processing to meet specific needs. However, it’s essential to note that CIFilter
objects are mutable and not thread-safe, so care must be taken when using them in multithreaded applications.
import CoreImage
// Load the input image
if let inputUIImage = UIImage(named: "image-example"),
let ciImage = CIImage(image: inputUIImage) {
// Use the Pixellate filter
if let filter = CIFilter(name: "CIPixellate") {
filter.setValue(ciImage, forKey: kCIInputImageKey)
filter.setValue(25.0, forKey: kCIInputScaleKey)
// Get the processed image
if let outputCIImage = filter.outputImage {
print("Successfully applied the Pixellate filter")
}
} else {
print("Failed to create the Pixellate filter")
}
}
Developers needed to know the names of the CIFilter
s they wanted to use. A comprehensive list of CIFilters can be found in the Core Image Filter Reference section of the Documentation Archive. To simplify the use of CIFilter
, Apple introduced in iOS13 the CoreImage.CIFilterBuiltins
module.
List of builtin filters
CICategoryBlur:
- CIBokehBlur
- CIBoxBlur
- CIDepthBlurEffect
- CIDiscBlur
- CIGaussianBlur
- CIMaskedVariableBlur
- CIMedianFilter
- CIMorphologyGradient
- CIMorphologyMaximum
- CIMorphologyMinimum
- CIMorphologyRectangleMaximum
- CIMorphologyRectangleMinimum
- CIMotionBlur
- CINoiseReduction
- CIZoomBlur
CICategoryBuiltIn:
- CIAccordionFoldTransition
- CIAdditionCompositing
- CIAffineClamp
- CIAffineTile
- CIAffineTransform
- CIAreaAlphaWeightedHistogram
- CIAreaAverage
- CIAreaBoundsRed
- CIAreaHistogram
- CIAreaLogarithmicHistogram
- CIAreaMaximum
- CIAreaMaximumAlpha
- CIAreaMinMax
- CIAreaMinMaxRed
- CIAreaMinimum
- CIAreaMinimumAlpha
- CIAttributedTextImageGenerator
- CIAztecCodeGenerator
- CIBarcodeGenerator
- CIBarsSwipeTransition
- CIBicubicScaleTransform
- CIBlendWithAlphaMask
- CIBlendWithBlueMask
- CIBlendWithMask
- CIBlendWithRedMask
- CIBloom
- CIBlurredRectangleGenerator
- CIBokehBlur
- CIBoxBlur
- CIBumpDistortion
- CIBumpDistortionLinear
- CICMYKHalftone
- CICameraCalibrationLensCorrection
- CICannyEdgeDetector
- CICheckerboardGenerator
- CICircleSplashDistortion
- CICircularScreen
- CICircularWrap
- CIClamp
- CICode128BarcodeGenerator
- CIColorAbsoluteDifference
- CIColorBlendMode
- CIColorBurnBlendMode
- CIColorClamp
- CIColorControls
- CIColorCrossPolynomial
- CIColorCube
- CIColorCubeWithColorSpace
- CIColorCubesMixedWithMask
- CIColorCurves
- CIColorDodgeBlendMode
- CIColorInvert
- CIColorMap
- CIColorMatrix
- CIColorMonochrome
- CIColorPolynomial
- CIColorPosterize
- CIColorThreshold
- CIColorThresholdOtsu
- CIColumnAverage
- CIComicEffect
- CIConstantColorGenerator
- CIConvertLabToRGB
- CIConvertRGBtoLab
- CIConvolution3X3
- CIConvolution5X5
- CIConvolution7X7
- CIConvolution9Horizontal
- CIConvolution9Vertical
- CIConvolutionRGB3X3
- CIConvolutionRGB5X5
- CIConvolutionRGB7X7
- CIConvolutionRGB9Horizontal
- CIConvolutionRGB9Vertical
- CICopyMachineTransition
- CICoreMLModelFilter
- CICrop
- CICrystallize
- CIDarkenBlendMode
- CIDepthBlurEffect
- CIDepthOfField
- CIDepthToDisparity
- CIDifferenceBlendMode
- CIDiscBlur
- CIDisintegrateWithMaskTransition
- CIDisparityToDepth
- CIDisplacementDistortion
- CIDissolveTransition
- CIDistanceGradientFromRedMask
- CIDither
- CIDivideBlendMode
- CIDocumentEnhancer
- CIDotScreen
- CIDroste
- CIEdgePreserveUpsampleFilter
- CIEdgeWork
- CIEdges
- CIEightfoldReflectedTile
- CIExclusionBlendMode
- CIExposureAdjust
- CIFalseColor
- CIFlashTransition
- CIFourfoldReflectedTile
- CIFourfoldRotatedTile
- CIFourfoldTranslatedTile
- CIGaborGradients
- CIGammaAdjust
- CIGaussianBlur
- CIGaussianGradient
- CIGlassDistortion
- CIGlassLozenge
- CIGlideReflectedTile
- CIGloom
- CIGuidedFilter
- CIHardLightBlendMode
- CIHatchedScreen
- CIHeightFieldFromMask
- CIHexagonalPixellate
- CIHighlightShadowAdjust
- CIHistogramDisplayFilter
- CIHoleDistortion
- CIHueAdjust
- CIHueBlendMode
- CIHueSaturationValueGradient
- CIKMeans
- CIKaleidoscope
- CIKeystoneCorrectionCombined
- CIKeystoneCorrectionHorizontal
- CIKeystoneCorrectionVertical
- CILabDeltaE
- CILanczosScaleTransform
- CILenticularHaloGenerator
- CILightTunnel
- CILightenBlendMode
- CILineOverlay
- CILineScreen
- CILinearBurnBlendMode
- CILinearDodgeBlendMode
- CILinearGradient
- CILinearLightBlendMode
- CILinearToSRGBToneCurve
- CILuminosityBlendMode
- CIMaskToAlpha
- CIMaskedVariableBlur
- CIMaximumComponent
- CIMaximumCompositing
- CIMaximumScaleTransform
- CIMedianFilter
- CIMeshGenerator
- CIMinimumComponent
- CIMinimumCompositing
- CIMix
- CIModTransition
- CIMorphologyGradient
- CIMorphologyMaximum
- CIMorphologyMinimum
- CIMorphologyRectangleMaximum
- CIMorphologyRectangleMinimum
- CIMotionBlur
- CIMultiplyBlendMode
- CIMultiplyCompositing
- CINinePartStretched
- CINinePartTiled
- CINoiseReduction
- CIOpTile
- CIOverlayBlendMode
- CIPDF417BarcodeGenerator
- CIPageCurlTransition
- CIPageCurlWithShadowTransition
- CIPaletteCentroid
- CIPalettize
- CIParallelogramTile
- CIPersonSegmentation
- CIPerspectiveCorrection
- CIPerspectiveRotate
- CIPerspectiveTile
- CIPerspectiveTransform
- CIPerspectiveTransformWithExtent
- CIPhotoEffectChrome
- CIPhotoEffectFade
- CIPhotoEffectInstant
- CIPhotoEffectMono
- CIPhotoEffectNoir
- CIPhotoEffectProcess
- CIPhotoEffectTonal
- CIPhotoEffectTransfer
- CIPinLightBlendMode
- CIPinchDistortion
- CIPixellate
- CIPointillize
- CIQRCodeGenerator
- CIRadialGradient
- CIRandomGenerator
- CIRippleTransition
- CIRoundedRectangleGenerator
- CIRoundedRectangleStrokeGenerator
- CIRowAverage
- CISRGBToneCurveToLinear
- CISaliencyMapFilter
- CISampleNearest
- CISaturationBlendMode
- CIScreenBlendMode
- CISepiaTone
- CIShadedMaterial
- CISharpenLuminance
- CISixfoldReflectedTile
- CISixfoldRotatedTile
- CISmoothLinearGradient
- CISobelGradients
- CISoftLightBlendMode
- CISourceAtopCompositing
- CISourceInCompositing
- CISourceOutCompositing
- CISourceOverCompositing
- CISpotColor
- CISpotLight
- CIStarShineGenerator
- CIStraightenFilter
- CIStretchCrop
- CIStripesGenerator
- CISubtractBlendMode
- CISunbeamsGenerator
- CISwipeTransition
- CITemperatureAndTint
- CITextImageGenerator
- CIThermal
- CIToneCurve
- CIToneMapHeadroom
- CITorusLensDistortion
- CITriangleKaleidoscope
- CITriangleTile
- CITwelvefoldReflectedTile
- CITwirlDistortion
- CIUnsharpMask
- CIVibrance
- CIVignette
- CIVignetteEffect
- CIVividLightBlendMode
- CIVortexDistortion
- CIWhitePointAdjust
- CIXRay
- CIZoomBlur
CICategoryColorAdjustment:
- CIColorAbsoluteDifference
- CIColorClamp
- CIColorControls
- CIColorMatrix
- CIColorPolynomial
- CIColorThreshold
- CIColorThresholdOtsu
- CIDepthToDisparity
- CIDisparityToDepth
- CIExposureAdjust
- CIGammaAdjust
- CIHueAdjust
- CILinearToSRGBToneCurve
- CISRGBToneCurveToLinear
- CITemperatureAndTint
- CIToneCurve
- CIToneMapHeadroom
- CIVibrance
- CIWhitePointAdjust
CICategoryColorEffect:
- CIColorCrossPolynomial
- CIColorCube
- CIColorCubeWithColorSpace
- CIColorCubesMixedWithMask
- CIColorCurves
- CIColorInvert
- CIColorMap
- CIColorMonochrome
- CIColorPosterize
- CIConvertLabToRGB
- CIConvertRGBtoLab
- CIDither
- CIDocumentEnhancer
- CIFalseColor
- CILabDeltaE
- CIMaskToAlpha
- CIMaximumComponent
- CIMinimumComponent
- CIPaletteCentroid
- CIPalettize
- CIPhotoEffectChrome
- CIPhotoEffectFade
- CIPhotoEffectInstant
- CIPhotoEffectMono
- CIPhotoEffectNoir
- CIPhotoEffectProcess
- CIPhotoEffectTonal
- CIPhotoEffectTransfer
- CISepiaTone
- CIThermal
- CIVignette
- CIVignetteEffect
- CIXRay
CICategoryCompositeOperation:
- CIAdditionCompositing
- CIColorBlendMode
- CIColorBurnBlendMode
- CIColorDodgeBlendMode
- CIDarkenBlendMode
- CIDifferenceBlendMode
- CIDivideBlendMode
- CIExclusionBlendMode
- CIHardLightBlendMode
- CIHueBlendMode
- CILightenBlendMode
- CILinearBurnBlendMode
- CILinearDodgeBlendMode
- CILinearLightBlendMode
- CILuminosityBlendMode
- CIMaximumCompositing
- CIMinimumCompositing
- CIMultiplyBlendMode
- CIMultiplyCompositing
- CIOverlayBlendMode
- CIPinLightBlendMode
- CISaturationBlendMode
- CIScreenBlendMode
- CISoftLightBlendMode
- CISourceAtopCompositing
- CISourceInCompositing
- CISourceOutCompositing
- CISourceOverCompositing
- CISubtractBlendMode
- CIVividLightBlendMode
CICategoryDistortionEffect:
- CIBumpDistortion
- CIBumpDistortionLinear
- CICameraCalibrationLensCorrection
- CICircleSplashDistortion
- CICircularWrap
- CIDisplacementDistortion
- CIDroste
- CIGlassDistortion
- CIGlassLozenge
- CIHoleDistortion
- CILightTunnel
- CINinePartStretched
- CINinePartTiled
- CIPinchDistortion
- CIStretchCrop
- CITorusLensDistortion
- CITwirlDistortion
- CIVortexDistortion
CICategoryGenerator:
- CIAttributedTextImageGenerator
- CIAztecCodeGenerator
- CIBarcodeGenerator
- CIBlurredRectangleGenerator
- CICheckerboardGenerator
- CICode128BarcodeGenerator
- CIConstantColorGenerator
- CILenticularHaloGenerator
- CIMeshGenerator
- CIPDF417BarcodeGenerator
- CIQRCodeGenerator
- CIRandomGenerator
- CIRoundedRectangleGenerator
- CIRoundedRectangleStrokeGenerator
- CIStarShineGenerator
- CIStripesGenerator
- CISunbeamsGenerator
- CITextImageGenerator
CICategoryGeometryAdjustment:
- CIAffineTransform
- CIBicubicScaleTransform
- CICrop
- CIEdgePreserveUpsampleFilter
- CIGuidedFilter
- CIKeystoneCorrectionCombined
- CIKeystoneCorrectionHorizontal
- CIKeystoneCorrectionVertical
- CILanczosScaleTransform
- CIMaximumScaleTransform
- CIPerspectiveCorrection
- CIPerspectiveRotate
- CIPerspectiveTransform
- CIPerspectiveTransformWithExtent
- CIStraightenFilter
CICategoryGradient:
- CIDistanceGradientFromRedMask
- CIGaussianGradient
- CIHueSaturationValueGradient
- CILinearGradient
- CIRadialGradient
- CISmoothLinearGradient
CICategoryHalftoneEffect:
- CICMYKHalftone
- CICircularScreen
- CIDotScreen
- CIHatchedScreen
- CILineScreen
CICategoryHighDynamicRange:
- CIAccordionFoldTransition
- CIAdditionCompositing
- CIAffineClamp
- CIAffineTile
- CIAffineTransform
- CIAreaAverage
- CIAreaBoundsRed
- CIAreaLogarithmicHistogram
- CIAreaMaximum
- CIAreaMaximumAlpha
- CIAreaMinMax
- CIAreaMinMaxRed
- CIAreaMinimum
- CIAreaMinimumAlpha
- CIBarsSwipeTransition
- CIBicubicScaleTransform
- CIBlendWithAlphaMask
- CIBlendWithBlueMask
- CIBlendWithMask
- CIBlendWithRedMask
- CIBloom
- CIBlurredRectangleGenerator
- CIBokehBlur
- CIBoxBlur
- CIBumpDistortion
- CIBumpDistortionLinear
- CICameraCalibrationLensCorrection
- CICannyEdgeDetector
- CICheckerboardGenerator
- CICircleSplashDistortion
- CICircularWrap
- CIClamp
- CIColorAbsoluteDifference
- CIColorControls
- CIColorCrossPolynomial
- CIColorCube
- CIColorCubeWithColorSpace
- CIColorCubesMixedWithMask
- CIColorInvert
- CIColorMatrix
- CIColorMonochrome
- CIColorPolynomial
- CIColorPosterize
- CIColumnAverage
- CIConstantColorGenerator
- CIConvertLabToRGB
- CIConvertRGBtoLab
- CIConvolution3X3
- CIConvolution5X5
- CIConvolution7X7
- CIConvolution9Horizontal
- CIConvolution9Vertical
- CIConvolutionRGB3X3
- CIConvolutionRGB5X5
- CIConvolutionRGB7X7
- CIConvolutionRGB9Horizontal
- CIConvolutionRGB9Vertical
- CICopyMachineTransition
- CICrop
- CICrystallize
- CIDepthBlurEffect
- CIDiscBlur
- CIDisintegrateWithMaskTransition
- CIDisplacementDistortion
- CIDissolveTransition
- CIDither
- CIDroste
- CIEdgePreserveUpsampleFilter
- CIEdges
- CIEightfoldReflectedTile
- CIExposureAdjust
- CIFalseColor
- CIFlashTransition
- CIFourfoldReflectedTile
- CIFourfoldRotatedTile
- CIFourfoldTranslatedTile
- CIGaborGradients
- CIGammaAdjust
- CIGaussianBlur
- CIGaussianGradient
- CIGlassDistortion
- CIGlassLozenge
- CIGlideReflectedTile
- CIGloom
- CIGuidedFilter
- CIHexagonalPixellate
- CIHighlightShadowAdjust
- CIHoleDistortion
- CIHueAdjust
- CIKMeans
- CIKaleidoscope
- CIKeystoneCorrectionCombined
- CIKeystoneCorrectionHorizontal
- CIKeystoneCorrectionVertical
- CILanczosScaleTransform
- CILenticularHaloGenerator
- CILightTunnel
- CILinearGradient
- CILinearToSRGBToneCurve
- CIMaskedVariableBlur
- CIMaximumComponent
- CIMaximumCompositing
- CIMaximumScaleTransform
- CIMedianFilter
- CIMeshGenerator
- CIMinimumComponent
- CIMinimumCompositing
- CIMix
- CIModTransition
- CIMorphologyGradient
- CIMorphologyMaximum
- CIMorphologyMinimum
- CIMorphologyRectangleMaximum
- CIMorphologyRectangleMinimum
- CIMotionBlur
- CIMultiplyCompositing
- CINinePartStretched
- CINinePartTiled
- CINoiseReduction
- CIOpTile
- CIPageCurlTransition
- CIPageCurlWithShadowTransition
- CIParallelogramTile
- CIPerspectiveCorrection
- CIPerspectiveRotate
- CIPerspectiveTile
- CIPerspectiveTransform
- CIPerspectiveTransformWithExtent
- CIPhotoEffectChrome
- CIPhotoEffectFade
- CIPhotoEffectInstant
- CIPhotoEffectMono
- CIPhotoEffectNoir
- CIPhotoEffectProcess
- CIPhotoEffectTonal
- CIPhotoEffectTransfer
- CIPinchDistortion
- CIPixellate
- CIPointillize
- CIRadialGradient
- CIRippleTransition
- CIRoundedRectangleGenerator
- CIRoundedRectangleStrokeGenerator
- CIRowAverage
- CISRGBToneCurveToLinear
- CISampleNearest
- CISepiaTone
- CIShadedMaterial
- CISharpenLuminance
- CISixfoldReflectedTile
- CISixfoldRotatedTile
- CISmoothLinearGradient
- CISobelGradients
- CISourceAtopCompositing
- CISourceInCompositing
- CISourceOutCompositing
- CISourceOverCompositing
- CISpotColor
- CISpotLight
- CIStarShineGenerator
- CIStraightenFilter
- CIStretchCrop
- CIStripesGenerator
- CISunbeamsGenerator
- CISwipeTransition
- CITemperatureAndTint
- CIToneCurve
- CIToneMapHeadroom
- CITorusLensDistortion
- CITriangleKaleidoscope
- CITriangleTile
- CITwelvefoldReflectedTile
- CITwirlDistortion
- CIUnsharpMask
- CIVibrance
- CIVignette
- CIVignetteEffect
- CIVortexDistortion
- CIWhitePointAdjust
- CIZoomBlur
CICategoryInterlaced:
- CIAdditionCompositing
- CIColorAbsoluteDifference
- CIColorBlendMode
- CIColorBurnBlendMode
- CIColorClamp
- CIColorControls
- CIColorCrossPolynomial
- CIColorCube
- CIColorCubeWithColorSpace
- CIColorCubesMixedWithMask
- CIColorCurves
- CIColorDodgeBlendMode
- CIColorInvert
- CIColorMap
- CIColorMatrix
- CIColorMonochrome
- CIColorPolynomial
- CIColorPosterize
- CIColorThreshold
- CIColorThresholdOtsu
- CIConvertLabToRGB
- CIConvertRGBtoLab
- CIDarkenBlendMode
- CIDifferenceBlendMode
- CIDissolveTransition
- CIDivideBlendMode
- CIEdgePreserveUpsampleFilter
- CIExclusionBlendMode
- CIExposureAdjust
- CIFalseColor
- CIGammaAdjust
- CIHardLightBlendMode
- CIHueAdjust
- CIHueBlendMode
- CILabDeltaE
- CILightenBlendMode
- CILinearBurnBlendMode
- CILinearDodgeBlendMode
- CILinearLightBlendMode
- CILinearToSRGBToneCurve
- CILuminosityBlendMode
- CIMaskToAlpha
- CIMaximumComponent
- CIMaximumCompositing
- CIMinimumComponent
- CIMinimumCompositing
- CIMultiplyBlendMode
- CIMultiplyCompositing
- CIOverlayBlendMode
- CIPhotoEffectChrome
- CIPhotoEffectFade
- CIPhotoEffectInstant
- CIPhotoEffectMono
- CIPhotoEffectNoir
- CIPhotoEffectProcess
- CIPhotoEffectTonal
- CIPhotoEffectTransfer
- CIPinLightBlendMode
- CISRGBToneCurveToLinear
- CISaturationBlendMode
- CIScreenBlendMode
- CISepiaTone
- CISoftLightBlendMode
- CISourceAtopCompositing
- CISourceInCompositing
- CISourceOutCompositing
- CISourceOverCompositing
- CISubtractBlendMode
- CITemperatureAndTint
- CIThermal
- CIToneCurve
- CIToneMapHeadroom
- CIVibrance
- CIVignette
- CIVignetteEffect
- CIVividLightBlendMode
- CIWhitePointAdjust
- CIXRay
CICategoryNonSquarePixels:
- CIAdditionCompositing
- CIBicubicScaleTransform
- CIColorAbsoluteDifference
- CIColorBlendMode
- CIColorBurnBlendMode
- CIColorClamp
- CIColorControls
- CIColorCrossPolynomial
- CIColorCube
- CIColorCubeWithColorSpace
- CIColorCubesMixedWithMask
- CIColorCurves
- CIColorDodgeBlendMode
- CIColorInvert
- CIColorMap
- CIColorMatrix
- CIColorMonochrome
- CIColorPolynomial
- CIColorPosterize
- CIColorThreshold
- CIColorThresholdOtsu
- CIConvertLabToRGB
- CIConvertRGBtoLab
- CIDarkenBlendMode
- CIDifferenceBlendMode
- CIDissolveTransition
- CIDivideBlendMode
- CIDocumentEnhancer
- CIEdgePreserveUpsampleFilter
- CIExclusionBlendMode
- CIExposureAdjust
- CIFalseColor
- CIGammaAdjust
- CIHardLightBlendMode
- CIHueAdjust
- CIHueBlendMode
- CILabDeltaE
- CILightenBlendMode
- CILinearBurnBlendMode
- CILinearDodgeBlendMode
- CILinearLightBlendMode
- CILinearToSRGBToneCurve
- CILuminosityBlendMode
- CIMaskToAlpha
- CIMaximumComponent
- CIMaximumCompositing
- CIMinimumComponent
- CIMinimumCompositing
- CIMultiplyBlendMode
- CIMultiplyCompositing
- CIOverlayBlendMode
- CIPhotoEffectChrome
- CIPhotoEffectFade
- CIPhotoEffectInstant
- CIPhotoEffectMono
- CIPhotoEffectNoir
- CIPhotoEffectProcess
- CIPhotoEffectTonal
- CIPhotoEffectTransfer
- CIPinLightBlendMode
- CISRGBToneCurveToLinear
- CISaturationBlendMode
- CIScreenBlendMode
- CISepiaTone
- CISoftLightBlendMode
- CISourceAtopCompositing
- CISourceInCompositing
- CISourceOutCompositing
- CISourceOverCompositing
- CISubtractBlendMode
- CITemperatureAndTint
- CIThermal
- CIToneCurve
- CIToneMapHeadroom
- CIVibrance
- CIVividLightBlendMode
- CIWhitePointAdjust
- CIXRay
CICategoryReduction:
- CIAreaAlphaWeightedHistogram
- CIAreaAverage
- CIAreaBoundsRed
- CIAreaHistogram
- CIAreaLogarithmicHistogram
- CIAreaMaximum
- CIAreaMaximumAlpha
- CIAreaMinMax
- CIAreaMinMaxRed
- CIAreaMinimum
- CIAreaMinimumAlpha
- CIColumnAverage
- CIHistogramDisplayFilter
- CIKMeans
- CIRowAverage
CICategorySharpen:
- CISharpenLuminance
- CIUnsharpMask
CICategoryStillImage:
- CIAccordionFoldTransition
- CIAdditionCompositing
- CIAffineClamp
- CIAffineTile
- CIAffineTransform
- CIAreaAlphaWeightedHistogram
- CIAreaAverage
- CIAreaBoundsRed
- CIAreaHistogram
- CIAreaLogarithmicHistogram
- CIAreaMaximum
- CIAreaMaximumAlpha
- CIAreaMinMax
- CIAreaMinMaxRed
- CIAreaMinimum
- CIAreaMinimumAlpha
- CIAttributedTextImageGenerator
- CIAztecCodeGenerator
- CIBarcodeGenerator
- CIBarsSwipeTransition
- CIBicubicScaleTransform
- CIBlendWithAlphaMask
- CIBlendWithBlueMask
- CIBlendWithMask
- CIBlendWithRedMask
- CIBloom
- CIBlurredRectangleGenerator
- CIBokehBlur
- CIBoxBlur
- CIBumpDistortion
- CIBumpDistortionLinear
- CICMYKHalftone
- CICameraCalibrationLensCorrection
- CICannyEdgeDetector
- CICheckerboardGenerator
- CICircleSplashDistortion
- CICircularScreen
- CICircularWrap
- CIClamp
- CICode128BarcodeGenerator
- CIColorAbsoluteDifference
- CIColorBlendMode
- CIColorBurnBlendMode
- CIColorClamp
- CIColorControls
- CIColorCrossPolynomial
- CIColorCube
- CIColorCubeWithColorSpace
- CIColorCubesMixedWithMask
- CIColorCurves
- CIColorDodgeBlendMode
- CIColorInvert
- CIColorMap
- CIColorMatrix
- CIColorMonochrome
- CIColorPolynomial
- CIColorPosterize
- CIColorThreshold
- CIColorThresholdOtsu
- CIColumnAverage
- CIComicEffect
- CIConstantColorGenerator
- CIConvertLabToRGB
- CIConvertRGBtoLab
- CIConvolution3X3
- CIConvolution5X5
- CIConvolution7X7
- CIConvolution9Horizontal
- CIConvolution9Vertical
- CIConvolutionRGB3X3
- CIConvolutionRGB5X5
- CIConvolutionRGB7X7
- CIConvolutionRGB9Horizontal
- CIConvolutionRGB9Vertical
- CICopyMachineTransition
- CICoreMLModelFilter
- CICrop
- CICrystallize
- CIDarkenBlendMode
- CIDepthBlurEffect
- CIDepthOfField
- CIDepthToDisparity
- CIDifferenceBlendMode
- CIDiscBlur
- CIDisintegrateWithMaskTransition
- CIDisparityToDepth
- CIDisplacementDistortion
- CIDissolveTransition
- CIDistanceGradientFromRedMask
- CIDither
- CIDivideBlendMode
- CIDocumentEnhancer
- CIDotScreen
- CIDroste
- CIEdgePreserveUpsampleFilter
- CIEdgeWork
- CIEdges
- CIEightfoldReflectedTile
- CIExclusionBlendMode
- CIExposureAdjust
- CIFalseColor
- CIFlashTransition
- CIFourfoldReflectedTile
- CIFourfoldRotatedTile
- CIFourfoldTranslatedTile
- CIGaborGradients
- CIGammaAdjust
- CIGaussianBlur
- CIGaussianGradient
- CIGlassDistortion
- CIGlassLozenge
- CIGlideReflectedTile
- CIGloom
- CIGuidedFilter
- CIHardLightBlendMode
- CIHatchedScreen
- CIHeightFieldFromMask
- CIHexagonalPixellate
- CIHighlightShadowAdjust
- CIHistogramDisplayFilter
- CIHoleDistortion
- CIHueAdjust
- CIHueBlendMode
- CIHueSaturationValueGradient
- CIKMeans
- CIKaleidoscope
- CIKeystoneCorrectionCombined
- CIKeystoneCorrectionHorizontal
- CIKeystoneCorrectionVertical
- CILabDeltaE
- CILanczosScaleTransform
- CILenticularHaloGenerator
- CILightTunnel
- CILightenBlendMode
- CILineOverlay
- CILineScreen
- CILinearBurnBlendMode
- CILinearDodgeBlendMode
- CILinearGradient
- CILinearLightBlendMode
- CILinearToSRGBToneCurve
- CILuminosityBlendMode
- CIMaskToAlpha
- CIMaskedVariableBlur
- CIMaximumComponent
- CIMaximumCompositing
- CIMaximumScaleTransform
- CIMedianFilter
- CIMeshGenerator
- CIMinimumComponent
- CIMinimumCompositing
- CIMix
- CIModTransition
- CIMorphologyGradient
- CIMorphologyMaximum
- CIMorphologyMinimum
- CIMorphologyRectangleMaximum
- CIMorphologyRectangleMinimum
- CIMotionBlur
- CIMultiplyBlendMode
- CIMultiplyCompositing
- CINinePartStretched
- CINinePartTiled
- CINoiseReduction
- CIOpTile
- CIOverlayBlendMode
- CIPDF417BarcodeGenerator
- CIPageCurlTransition
- CIPageCurlWithShadowTransition
- CIPaletteCentroid
- CIPalettize
- CIParallelogramTile
- CIPersonSegmentation
- CIPerspectiveCorrection
- CIPerspectiveRotate
- CIPerspectiveTile
- CIPerspectiveTransform
- CIPerspectiveTransformWithExtent
- CIPhotoEffectChrome
- CIPhotoEffectFade
- CIPhotoEffectInstant
- CIPhotoEffectMono
- CIPhotoEffectNoir
- CIPhotoEffectProcess
- CIPhotoEffectTonal
- CIPhotoEffectTransfer
- CIPinLightBlendMode
- CIPinchDistortion
- CIPixellate
- CIPointillize
- CIQRCodeGenerator
- CIRadialGradient
- CIRandomGenerator
- CIRippleTransition
- CIRoundedRectangleGenerator
- CIRoundedRectangleStrokeGenerator
- CIRowAverage
- CISRGBToneCurveToLinear
- CISaliencyMapFilter
- CISampleNearest
- CISaturationBlendMode
- CIScreenBlendMode
- CISepiaTone
- CIShadedMaterial
- CISharpenLuminance
- CISixfoldReflectedTile
- CISixfoldRotatedTile
- CISmoothLinearGradient
- CISobelGradients
- CISoftLightBlendMode
- CISourceAtopCompositing
- CISourceInCompositing
- CISourceOutCompositing
- CISourceOverCompositing
- CISpotColor
- CISpotLight
- CIStarShineGenerator
- CIStraightenFilter
- CIStretchCrop
- CIStripesGenerator
- CISubtractBlendMode
- CISunbeamsGenerator
- CISwipeTransition
- CITemperatureAndTint
- CITextImageGenerator
- CIThermal
- CIToneCurve
- CIToneMapHeadroom
- CITorusLensDistortion
- CITriangleKaleidoscope
- CITriangleTile
- CITwelvefoldReflectedTile
- CITwirlDistortion
- CIUnsharpMask
- CIVibrance
- CIVignette
- CIVignetteEffect
- CIVividLightBlendMode
- CIVortexDistortion
- CIWhitePointAdjust
- CIXRay
- CIZoomBlur
CICategoryStylize:
- CIBlendWithAlphaMask
- CIBlendWithBlueMask
- CIBlendWithMask
- CIBlendWithRedMask
- CIBloom
- CICannyEdgeDetector
- CIComicEffect
- CIConvolution3X3
- CIConvolution5X5
- CIConvolution7X7
- CIConvolution9Horizontal
- CIConvolution9Vertical
- CIConvolutionRGB3X3
- CIConvolutionRGB5X5
- CIConvolutionRGB7X7
- CIConvolutionRGB9Horizontal
- CIConvolutionRGB9Vertical
- CICoreMLModelFilter
- CICrystallize
- CIDepthOfField
- CIEdgeWork
- CIEdges
- CIGaborGradients
- CIGloom
- CIHeightFieldFromMask
- CIHexagonalPixellate
- CIHighlightShadowAdjust
- CILineOverlay
- CIMix
- CIPersonSegmentation
- CIPixellate
- CIPointillize
- CISaliencyMapFilter
- CISampleNearest
- CIShadedMaterial
- CISobelGradients
- CISpotColor
- CISpotLight
CICategoryTileEffect:
- CIAffineClamp
- CIAffineTile
- CIClamp
- CIEightfoldReflectedTile
- CIFourfoldReflectedTile
- CIFourfoldRotatedTile
- CIFourfoldTranslatedTile
- CIGlideReflectedTile
- CIKaleidoscope
- CIOpTile
- CIParallelogramTile
- CIPerspectiveTile
- CISixfoldReflectedTile
- CISixfoldRotatedTile
- CITriangleKaleidoscope
- CITriangleTile
- CITwelvefoldReflectedTile
CICategoryTransition:
- CIAccordionFoldTransition
- CIBarsSwipeTransition
- CICopyMachineTransition
- CIDisintegrateWithMaskTransition
- CIDissolveTransition
- CIFlashTransition
- CIModTransition
- CIPageCurlTransition
- CIPageCurlWithShadowTransition
- CIRippleTransition
- CISwipeTransition
CICategoryVideo:
- CIAccordionFoldTransition
- CIAdditionCompositing
- CIAffineClamp
- CIAffineTile
- CIAffineTransform
- CIAreaAlphaWeightedHistogram
- CIAreaAverage
- CIAreaBoundsRed
- CIAreaHistogram
- CIAreaLogarithmicHistogram
- CIAreaMaximum
- CIAreaMaximumAlpha
- CIAreaMinMax
- CIAreaMinMaxRed
- CIAreaMinimum
- CIAreaMinimumAlpha
- CIAttributedTextImageGenerator
- CIBarcodeGenerator
- CIBarsSwipeTransition
- CIBicubicScaleTransform
- CIBlendWithAlphaMask
- CIBlendWithBlueMask
- CIBlendWithMask
- CIBlendWithRedMask
- CIBloom
- CIBokehBlur
- CIBoxBlur
- CIBumpDistortion
- CIBumpDistortionLinear
- CICMYKHalftone
- CICameraCalibrationLensCorrection
- CICannyEdgeDetector
- CICheckerboardGenerator
- CICircleSplashDistortion
- CICircularScreen
- CICircularWrap
- CIClamp
- CIColorAbsoluteDifference
- CIColorBlendMode
- CIColorBurnBlendMode
- CIColorClamp
- CIColorControls
- CIColorCrossPolynomial
- CIColorCube
- CIColorCubeWithColorSpace
- CIColorCubesMixedWithMask
- CIColorCurves
- CIColorDodgeBlendMode
- CIColorInvert
- CIColorMap
- CIColorMatrix
- CIColorMonochrome
- CIColorPolynomial
- CIColorPosterize
- CIColorThreshold
- CIColorThresholdOtsu
- CIColumnAverage
- CIComicEffect
- CIConstantColorGenerator
- CIConvertLabToRGB
- CIConvertRGBtoLab
- CIConvolution3X3
- CIConvolution5X5
- CIConvolution7X7
- CIConvolution9Horizontal
- CIConvolution9Vertical
- CIConvolutionRGB3X3
- CIConvolutionRGB5X5
- CIConvolutionRGB7X7
- CIConvolutionRGB9Horizontal
- CIConvolutionRGB9Vertical
- CICopyMachineTransition
- CICrop
- CICrystallize
- CIDarkenBlendMode
- CIDepthBlurEffect
- CIDepthOfField
- CIDepthToDisparity
- CIDifferenceBlendMode
- CIDiscBlur
- CIDisintegrateWithMaskTransition
- CIDisparityToDepth
- CIDisplacementDistortion
- CIDissolveTransition
- CIDistanceGradientFromRedMask
- CIDither
- CIDivideBlendMode
- CIDotScreen
- CIDroste
- CIEdgePreserveUpsampleFilter
- CIEdgeWork
- CIEdges
- CIEightfoldReflectedTile
- CIExclusionBlendMode
- CIExposureAdjust
- CIFalseColor
- CIFlashTransition
- CIFourfoldReflectedTile
- CIFourfoldRotatedTile
- CIFourfoldTranslatedTile
- CIGaborGradients
- CIGammaAdjust
- CIGaussianBlur
- CIGaussianGradient
- CIGlassDistortion
- CIGlassLozenge
- CIGlideReflectedTile
- CIGloom
- CIGuidedFilter
- CIHardLightBlendMode
- CIHatchedScreen
- CIHeightFieldFromMask
- CIHexagonalPixellate
- CIHighlightShadowAdjust
- CIHistogramDisplayFilter
- CIHoleDistortion
- CIHueAdjust
- CIHueBlendMode
- CIHueSaturationValueGradient
- CIKMeans
- CIKaleidoscope
- CIKeystoneCorrectionCombined
- CIKeystoneCorrectionHorizontal
- CIKeystoneCorrectionVertical
- CILabDeltaE
- CILanczosScaleTransform
- CILenticularHaloGenerator
- CILightTunnel
- CILightenBlendMode
- CILineOverlay
- CILineScreen
- CILinearBurnBlendMode
- CILinearDodgeBlendMode
- CILinearGradient
- CILinearLightBlendMode
- CILinearToSRGBToneCurve
- CILuminosityBlendMode
- CIMaskToAlpha
- CIMaskedVariableBlur
- CIMaximumComponent
- CIMaximumCompositing
- CIMaximumScaleTransform
- CIMedianFilter
- CIMeshGenerator
- CIMinimumComponent
- CIMinimumCompositing
- CIMix
- CIModTransition
- CIMorphologyGradient
- CIMorphologyMaximum
- CIMorphologyMinimum
- CIMorphologyRectangleMaximum
- CIMorphologyRectangleMinimum
- CIMotionBlur
- CIMultiplyBlendMode
- CIMultiplyCompositing
- CINinePartStretched
- CINinePartTiled
- CINoiseReduction
- CIOpTile
- CIOverlayBlendMode
- CIPDF417BarcodeGenerator
- CIPageCurlTransition
- CIPageCurlWithShadowTransition
- CIPaletteCentroid
- CIPalettize
- CIParallelogramTile
- CIPersonSegmentation
- CIPerspectiveCorrection
- CIPerspectiveRotate
- CIPerspectiveTile
- CIPerspectiveTransform
- CIPerspectiveTransformWithExtent
- CIPhotoEffectChrome
- CIPhotoEffectFade
- CIPhotoEffectInstant
- CIPhotoEffectMono
- CIPhotoEffectNoir
- CIPhotoEffectProcess
- CIPhotoEffectTonal
- CIPhotoEffectTransfer
- CIPinLightBlendMode
- CIPinchDistortion
- CIPixellate
- CIPointillize
- CIRadialGradient
- CIRandomGenerator
- CIRippleTransition
- CIRowAverage
- CISRGBToneCurveToLinear
- CISaliencyMapFilter
- CISampleNearest
- CISaturationBlendMode
- CIScreenBlendMode
- CISepiaTone
- CIShadedMaterial
- CISharpenLuminance
- CISixfoldReflectedTile
- CISixfoldRotatedTile
- CISmoothLinearGradient
- CISobelGradients
- CISoftLightBlendMode
- CISourceAtopCompositing
- CISourceInCompositing
- CISourceOutCompositing
- CISourceOverCompositing
- CISpotColor
- CISpotLight
- CIStarShineGenerator
- CIStraightenFilter
- CIStretchCrop
- CIStripesGenerator
- CISubtractBlendMode
- CISunbeamsGenerator
- CISwipeTransition
- CITemperatureAndTint
- CITextImageGenerator
- CIThermal
- CIToneCurve
- CIToneMapHeadroom
- CITorusLensDistortion
- CITriangleKaleidoscope
- CITriangleTile
- CITwelvefoldReflectedTile
- CITwirlDistortion
- CIUnsharpMask
- CIVibrance
- CIVignette
- CIVignetteEffect
- CIVividLightBlendMode
- CIVortexDistortion
- CIWhitePointAdjust
- CIXRay
- CIZoomBlur
CICategoryXMPSerializable:
- CIExposureAdjust
- CIPhotoEffectChrome
- CIPhotoEffectFade
- CIPhotoEffectInstant
- CIPhotoEffectMono
- CIPhotoEffectNoir
- CIPhotoEffectProcess
- CIPhotoEffectTonal
- CIPhotoEffectTransfer
- CISepiaTone
This provides a type-safe way to work with filters, making it easier to access and configure them without relying on key-value coding preventing runtime errors caused by incorrect parameter names or types.
import CoreImage
import CoreImage.CIFilterBuiltins
// Load the input image
if let inputUIImage = UIImage(named: "image-example"),
let ciImage = CIImage(image: inputUIImage) {
let filter = CIFilter.pixellate()
filter.inputImage = ciImage
filter.scale = 25.0
if let outputCIImage = filter.outputImage {
print("Successfully applied the Pixellate filter using CIFilterBuiltins")
}
}
CIContext
While CIImage
and CIFilter
define the processing instructions and transformations, CIContext
performs the actual rendering. It applies the filters and transformations generating the final output image from a CIImage
. The most common use case is a processed CIImage
being converted to a CGImage
for display or further manipulation.
import CoreImage
if let inputUIImage = UIImage(named: "image-example"),
let ciImage = CIImage(image: inputUIImage) {
let context = CIContext()
let filter = CIFilter.pixellate()
filter.inputImage = ciImage
filter.scale = 25.0
if let outputImage = filter.outputImage,
let cgImage = context.createCGImage(outputImage, from: outputImage.extent) {
let processedImage = UIImage(cgImage: cgImage)
print("Successfully rendered the image")
}
}
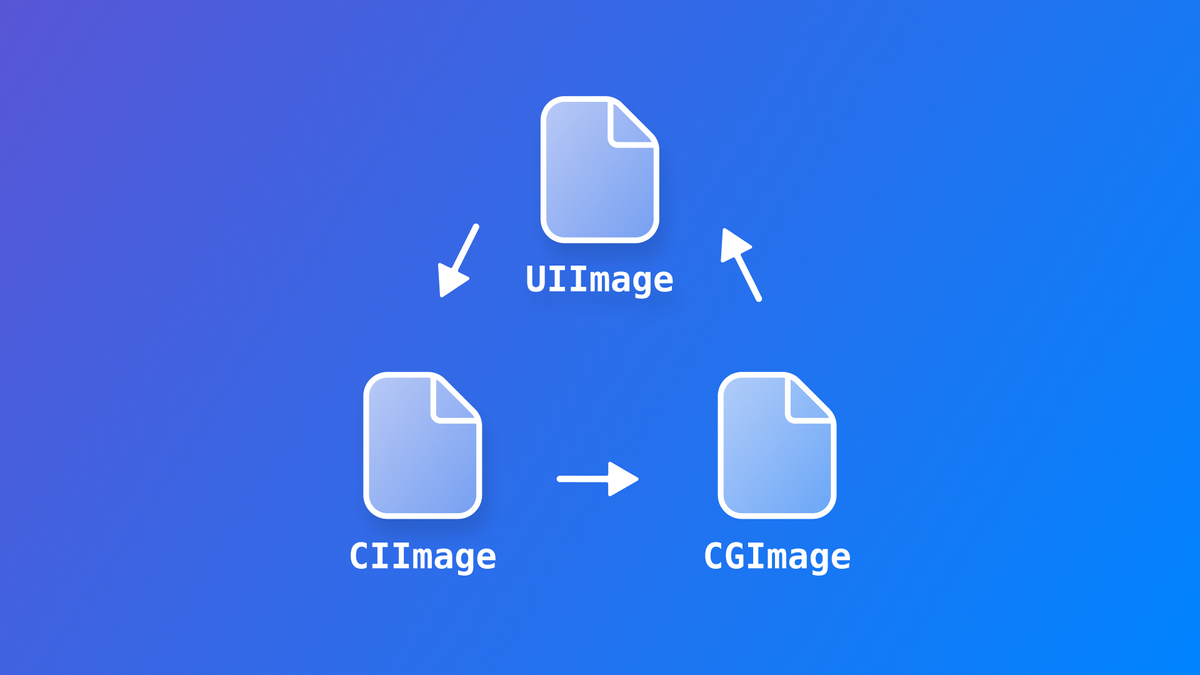
Or it can be used to render to a CVPixelBuffer
, really useful for real-time video processing.
import CoreImage
var pixelBuffer: CVPixelBuffer?
CVPixelBufferCreate(nil, 1920, 1080, kCVPixelFormatType_32BGRA, nil, &pixelBuffer)
let context = CIContext()
if let buffer = pixelBuffer {
let ciImage = CIImage(image: UIImage(named: "image-example")!)!
context.render(ciImage, to: buffer)
print("Rendered to pixel buffer successfully")
}
Core Image’s architecture is reflected in CIContext
. It can harness hardware acceleration via the GPU (using Metal or OpenGL) for high-performance tasks or gracefully fall back to the CPU for broader compatibility. You can in fact initialize a CIContext
in several ways, depending on your application needs.
// Basic CIContext
let basicContext = CIContext()
// GPU-Based CIContext (Metal)
if let device = MTLCreateSystemDefaultDevice() {
let gpuContext = CIContext(mtlDevice: device)
print("Created GPU-based CIContext")
}
// CPU-Based CIContext
let cpuContext = CIContext(options: [.useSoftwareRenderer: true])
print("Created CPU-based CIContext")
// Customizing Options
let customContext = CIContext(options: [
.workingColorSpace: CGColorSpaceCreateDeviceRGB(),
.highQualityDownsample: true
])
print("Created CIContext with custom options")
Reusing instances of CIContext
is critical for performance because creating one is relatively expensive in terms of resource allocation and initialization time. Each time a new context is created, it incurs overhead that can slow down image processing, especially in applications that require real-time performance, such as video processing or interactive image editing. When working with multiple images or frames, the cost of creating contexts repeatedly can lead to noticeable performance degradation. Developers should try to reuse the context as much as possible throughout the app instead of creating new ones.
You use CIContext
objects in conjunction with other Core Image classes, such as CIFilter
, CIImage
, and CIColor
, to process images using Core Image filters. You also use a Core Image context with theCIDetector
class to analyze images—for example, to detect faces or barcodes.
Simple Usage
Let’s see how to use Core Image within a SwiftUI application to apply a pixelated effect to an image.
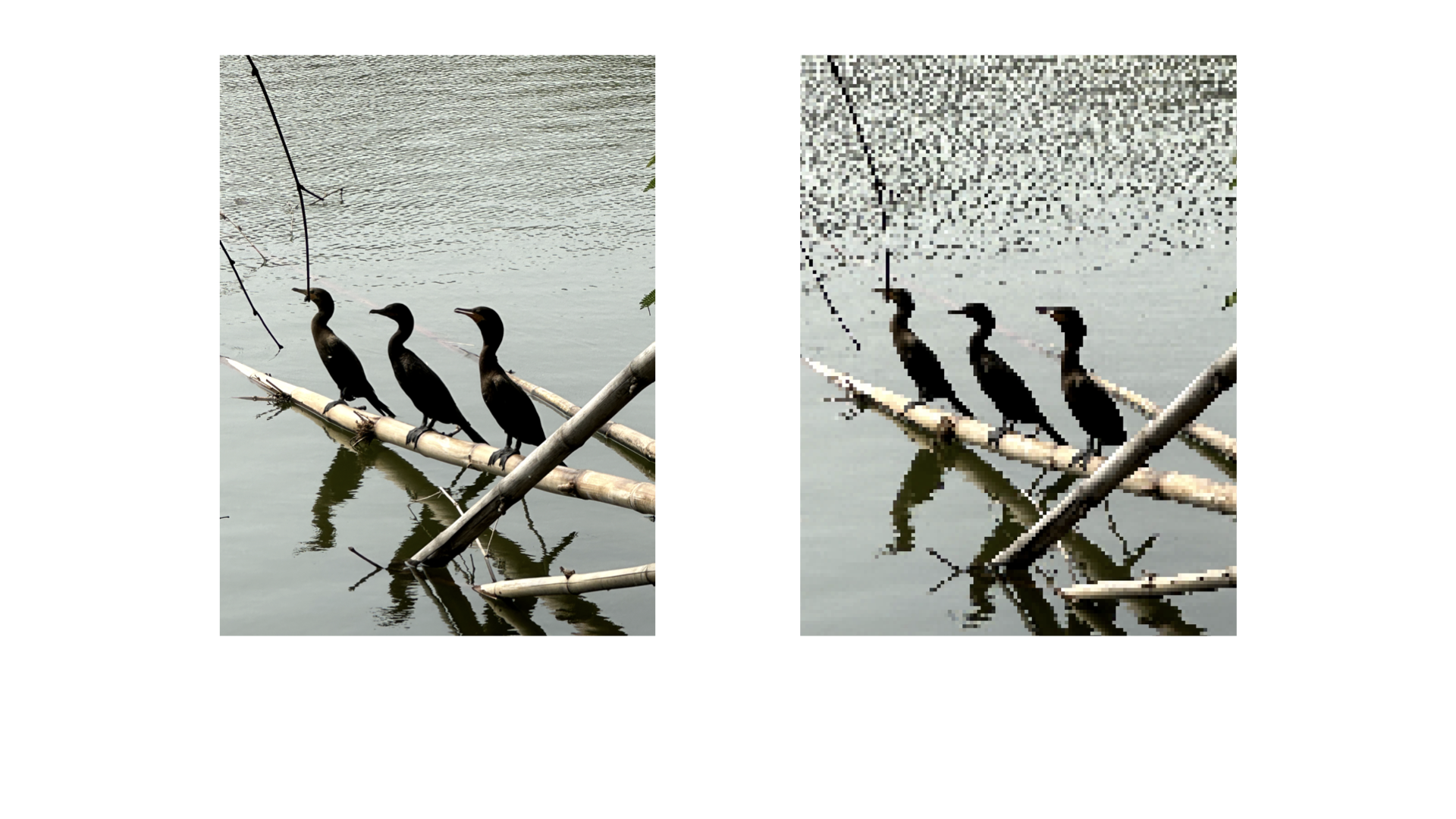
In this example, the users can select an image, apply a CIFilter.pixellate()
filter, and save the processed image to their photo library.
Here’s the code of the application:
import SwiftUI
import PhotosUI
import Photos
import CoreImage
import CoreImage.CIFilterBuiltins
struct ContentView: View {
@State private var inputImage: UIImage?
@State private var processedImage: UIImage?
@State private var imageSelection: PhotosPickerItem?
@State private var showAlert = false
@State private var alertMessage = ""
var body: some View {
NavigationStack {
VStack(spacing: 20) {
imageView
Spacer()
buttonsView
}
.padding()
.navigationTitle("Pixellated")
.alert(alertMessage, isPresented: $showAlert) {
Button("OK", role: .cancel) { }
}
}
.onChange(of: imageSelection) { _, newItem in
loadImage(from: newItem)
}
}
private var imageView: some View {
Group {
if let image = processedImage ?? inputImage {
Image(uiImage: image)
.resizable()
.scaledToFit()
.shadow(radius: 5)
.padding()
} else {
ContentUnavailableView("No Image Selected",
systemImage: "photo.badge.plus",
description: Text("Tap below to select a photo"))
}
}
}
private var buttonsView: some View {
VStack(spacing: 16) {
HStack(spacing: 12) {
if processedImage == nil {
Button("Apply Effect") {
applyEffect()
}
.buttonStyle(.borderedProminent)
.disabled(inputImage == nil)
.frame(maxWidth: .infinity)
}
PhotosPicker(selection: $imageSelection,
matching: .images) {
Text(inputImage == nil ? "Select Photo" : "Change Photo")
}
.buttonStyle(.bordered)
.frame(maxWidth: .infinity)
}
.controlSize(.large)
if processedImage != nil {
HStack(spacing: 12) {
Button("Reset") {
processedImage = nil
}
.buttonStyle(.bordered)
.frame(maxWidth: .infinity)
Button("Save") {
saveImage()
}
.buttonStyle(.borderedProminent)
.frame(maxWidth: .infinity)
}
.controlSize(.large)
}
}
.padding(.horizontal)
}
private func loadImage(from item: PhotosPickerItem?) {
Task {
if let data = try? await item?.loadTransferable(type: Data.self),
let uiImage = UIImage(data: data) {
inputImage = uiImage
processedImage = nil
}
}
}
private func applyEffect() {
guard let inputImage,
let ciImage = CIImage(image: inputImage) else { return }
let context = CIContext()
let filter = CIFilter.pixellate()
filter.inputImage = ciImage
filter.scale = 200.0
if let outputImage = filter.outputImage,
let cgImage = context.createCGImage(outputImage, from: outputImage.extent) {
processedImage = UIImage(cgImage: cgImage,
scale: inputImage.scale,
orientation: inputImage.imageOrientation)
}
}
private func saveImage() {
guard let processedImage else { return }
PHPhotoLibrary.requestAuthorization(for: .addOnly) { status in
guard status == .authorized else {
DispatchQueue.main.async {
alertMessage = "Failed to Save Image"
showAlert = true
}
return
}
PHPhotoLibrary.shared().performChanges {
PHAssetChangeRequest.creationRequestForAsset(from: processedImage)
} completionHandler: { success, _ in
DispatchQueue.main.async {
alertMessage = success ? "Image Saved Successfully" : "Failed to Save Image"
showAlert = true
}
}
}
}
}
The example leverages the CIFilterBuiltins
module to simplify working with Core Image filters in Swift. The CIFilter.pixellate()
filter is used to modify a CIImage
by applying a pixelation effect. The intensity of the effect is controlled by adjusting the scale property of the filter.
Once the filter is applied, a CIContext
is used to render the processed CIImage
into a CGImage
. The CIContext
serves as the rendering engine for converting Core Image objects into native graphics objects. Finally, the resulting CGImage
is converted into a UIImage
, which can then be displayed in the SwiftUI view.
private func applyEffect() {
// Ensure that an input image exists and convert it to a CIImage
guard let inputImage,
let ciImage = CIImage(image: inputImage) else { return }
// Create a Core Image context, which is responsible for rendering CIImage objects
let context = CIContext()
// Create a pixellate filter using CIFilterBuiltins
let filter = CIFilter.pixellate()
// Assign the input CIImage to the filter
filter.inputImage = ciImage
// Set the scale property to control the intensity of the pixelation
filter.scale = 200.0
// Apply the filter and attempt to render the output image
if let outputImage = filter.outputImage,
// Convert the processed CIImage into a CGImage for further use
let cgImage = context.createCGImage(outputImage, from: outputImage.extent) {
// Convert the CGImage into a UIImage to display in the SwiftUI view
processedImage = UIImage(cgImage: cgImage,
scale: inputImage.scale,
orientation: inputImage.imageOrientation)
}
}
Conclusion
Core Image is a versatile and powerful framework that empowers developers to perform advanced image processing efficiently. Its architecture provides a robust foundation for creating real-time visual effects, enhancing images, and performing complex transformations ensuring high performance across a variety of devices and scenarios. With its rich set of built-in filters, customizable processing pipeline, and seamless integration with Apple’s ecosystem, Core Image is a cornerstone of modern image processing on iOS and macOS platforms.