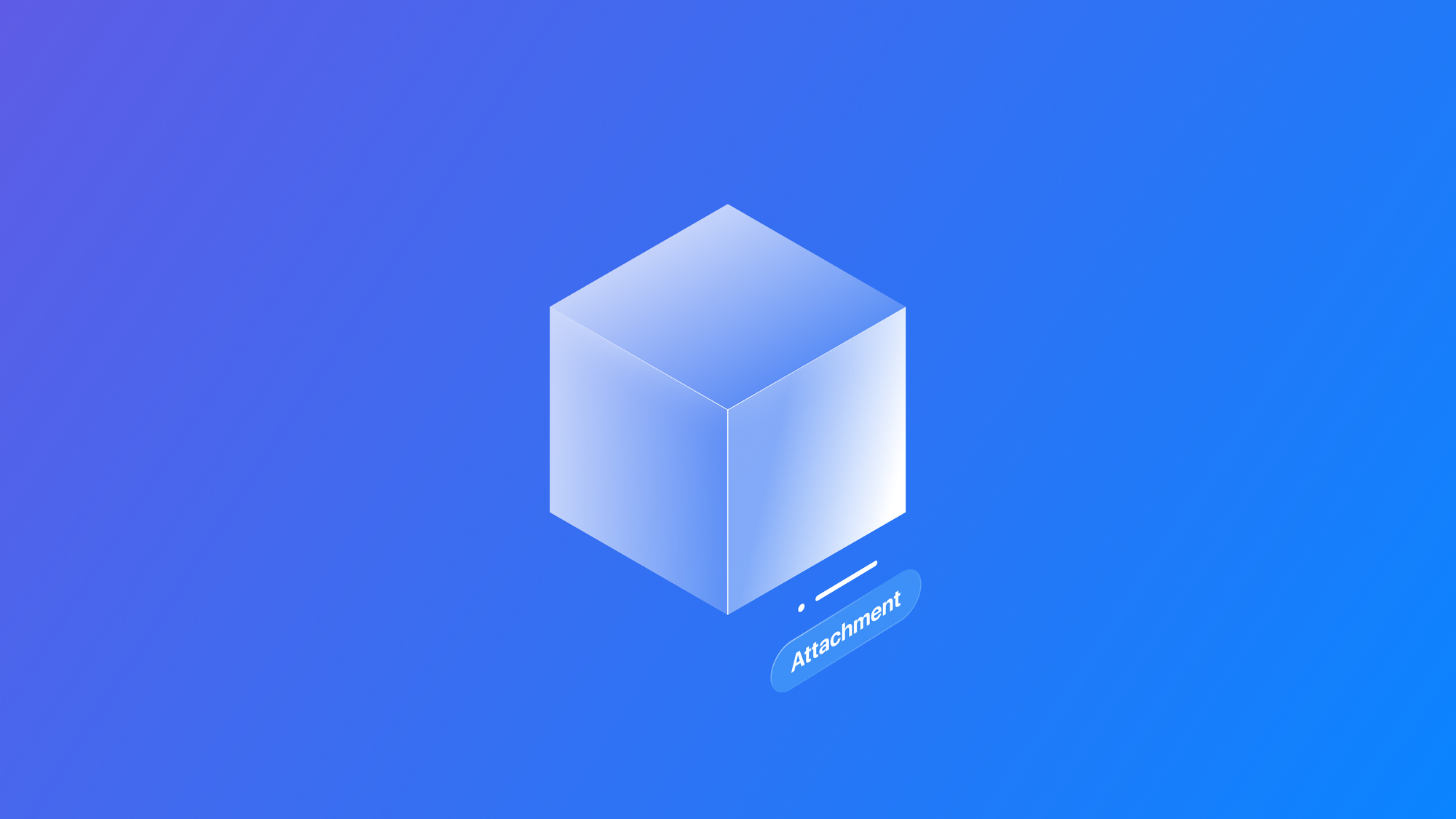
Using Attachments in a RealityView
Learn how to display SwiftUI views in a RealityView for visionOS applications.
RealityView
has a unique feature that allows you to integrate SwiftUI views into your RealityKit content. Attachments are views that can be positioned at specific locations relative to your RealityKit entities.
To display an Attachment
in a RealityView
use the following initializer init(make:update:placeholder:attachments:)
.
RealityView { content, attachments in
if let glassCube = try? await Entity(named: "GlassCube") {
content.add(glassCube)
//3. Retrieve the attachment with the "GlassCubeLabel" identifier as an entity.
if let glassCubeAttachment = attachments.entity(for: "GlassCubeLabel") {
//4. Position the Attachment and add it to the RealityViewContent
glassCubeAttachment.position = [0, -0.1, 0]
glassCube.addChild(glassCubeAttachment)
}
}
} placeholder: {
ProgressView()
} attachments: {
//1. Create the Attachment
Attachment(id: "GlassCubeLabel") {
//2. Define the SwiftUI View
Text("Glass Cube")
.font(.extraLargeTitle)
.padding()
.glassBackgroundEffect()
}
}
Let's go through the example code above.
In the attachments
closure an Attachment
object is created with an identifier (in the example the identifier is GlassCubeLabel). The content of an Attachment
object defines the SwiftUI view that will be displayed with the RealityView
content.
An attachment view is not automatically added to the content of a RealityView
. In the make
closure, where you define the entities displayed, you can have access to the attachment objects, theRealityViewAttachments
, and with entity(for:)
you can find a specific attachment with an identifier. The attachment will then be returned as a ViewAttachmentEntity
object.
Our entity, named glassCubeAttachment
, can now be added to the RealityView
as a child of the glassCube
entity.
By doing so, the attachment will be positioned always in the same position relative to the glass cube even when it is moved.
Positioning an attachment
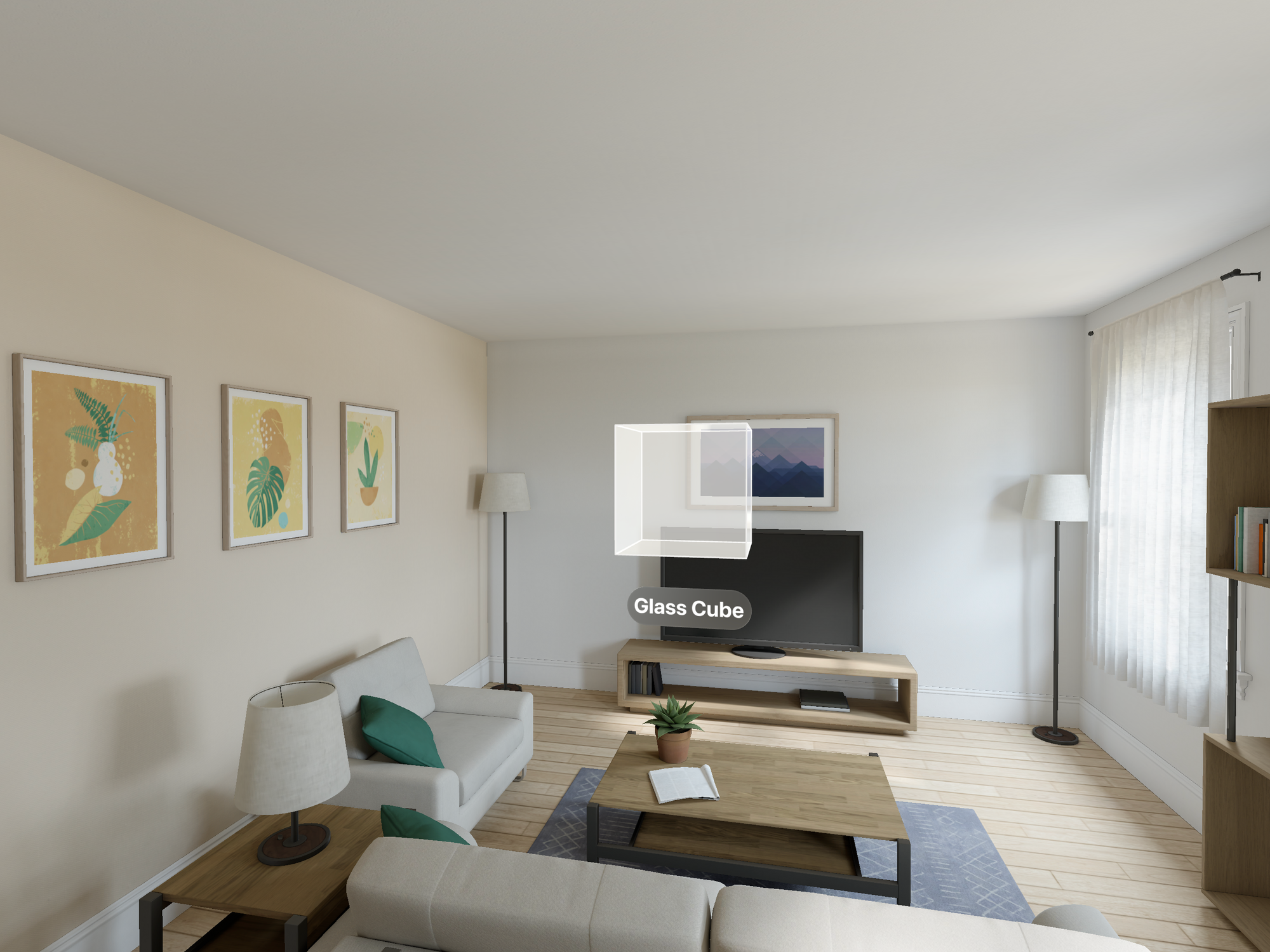
By default, an attachment will be placed at the origin of its parent entity. It is important to position it correctly.
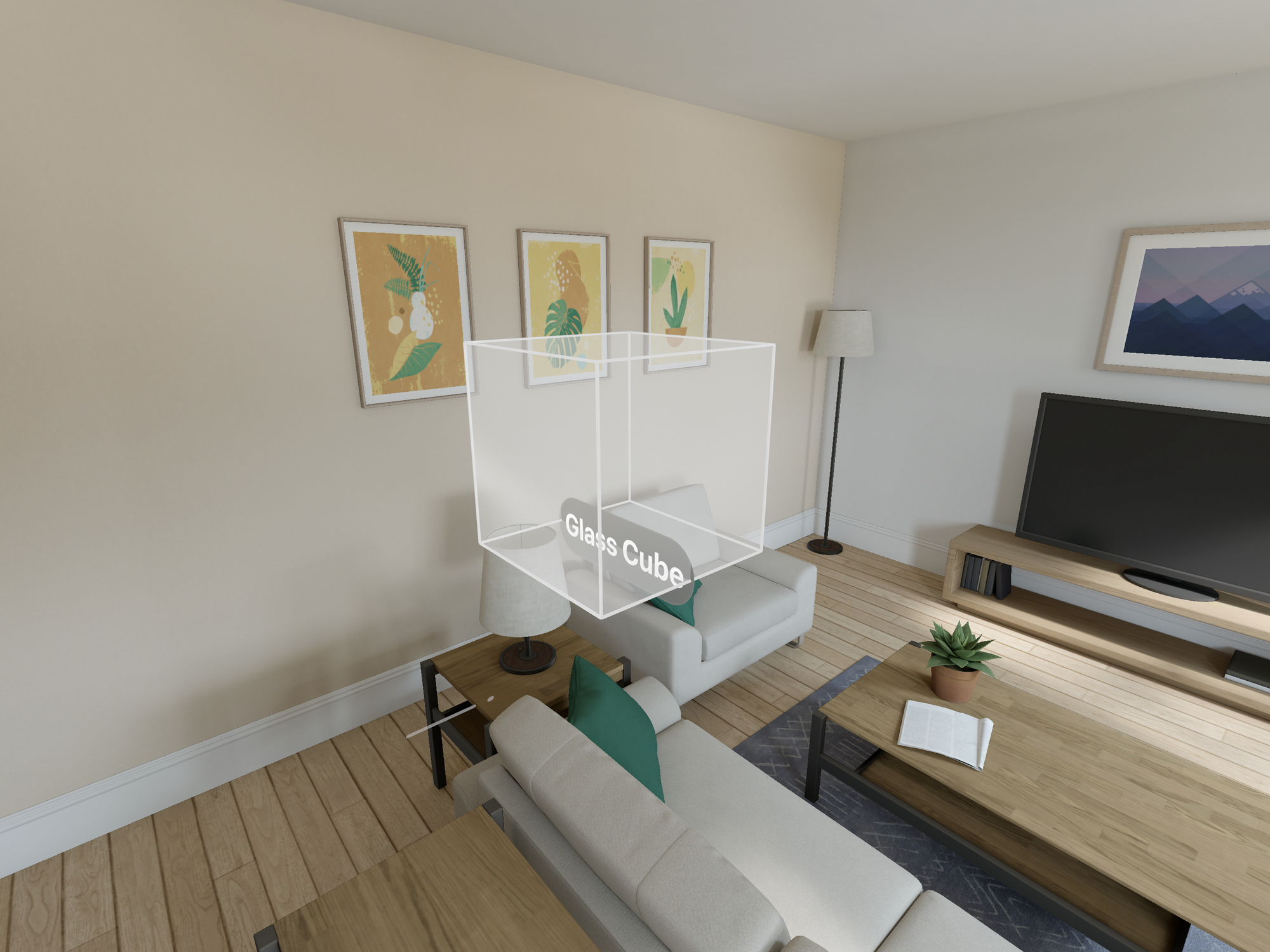
When placing attachments it is important to remember that the coordinate system in RealityView
has a different unit and y-axis orientation compared to SwiftUI. The y-axis points upwards and a 1 point represents 1 meter.
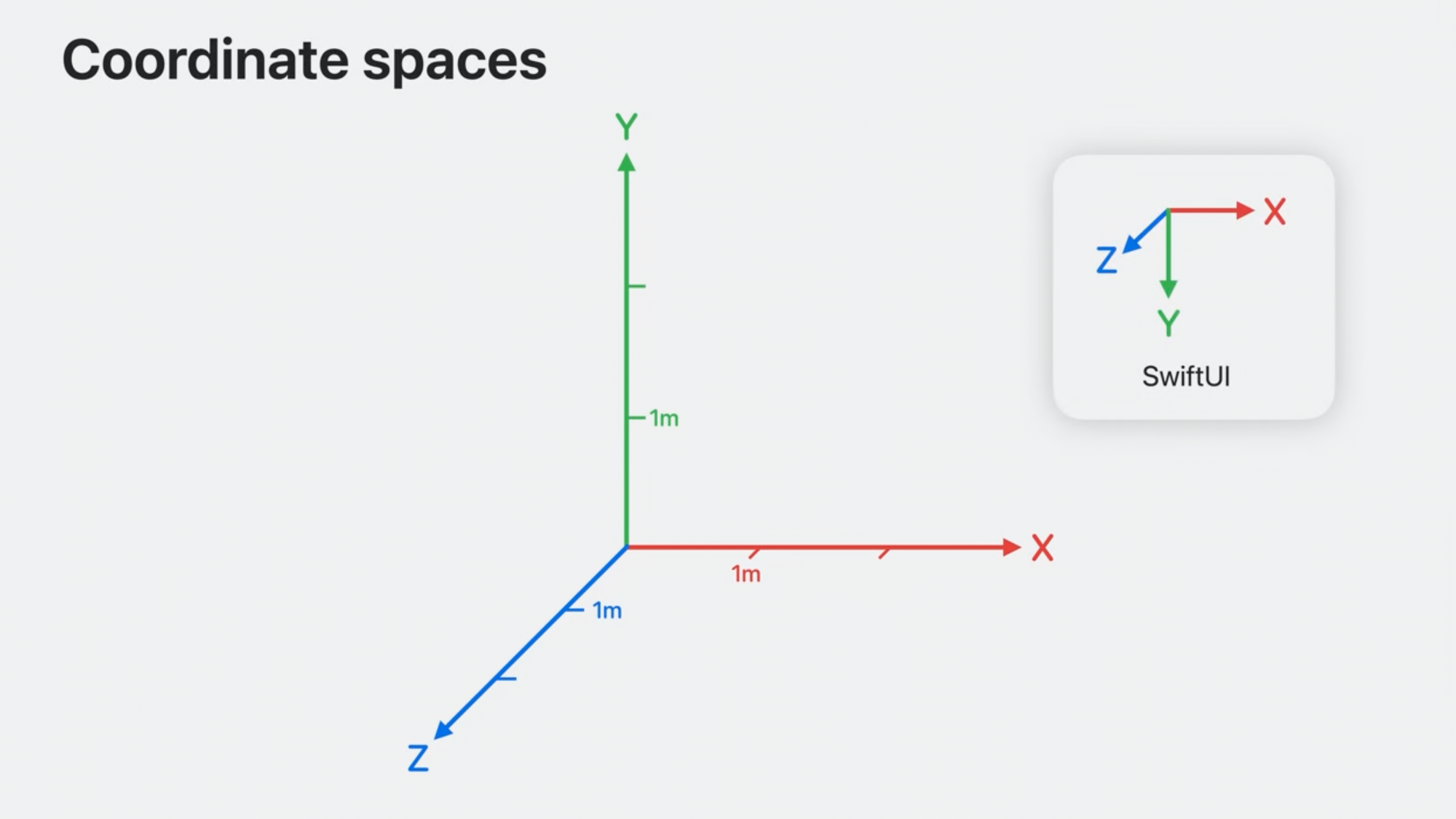
Keep in mind
Most of the APIs discussed in this article are still in beta and may be subject to changes in the future.
For example, in the Enhance your spatial computing app with RealityKit WWDC23 video the approach to display an attachment in a RealityView
is by using the .tag(_:)
modifier to reference the attachment entity in the make closure. This method is not working anymore and generates the following error: