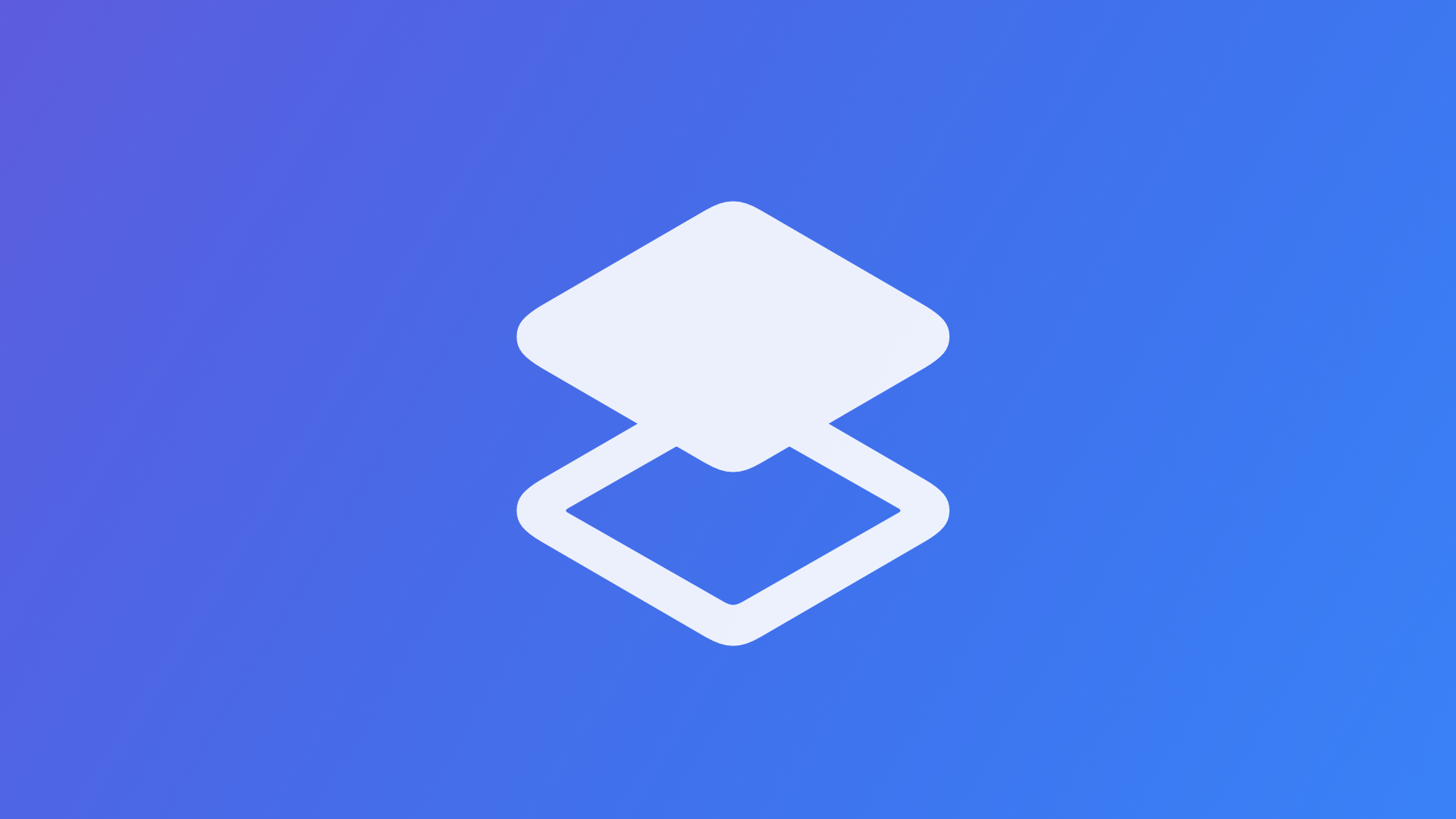
Using MapPolygon overlays in MapKit with SwiftUI
Learn how to place polygonal overlays on a Map view in SwiftUI.
When using MapKit with SwiftUI adding overlays on the map is quite simple.
Map overlays are shapes rendered on top of the map and they come in different types:
A MapPolygon
is a closed polygon overlay that is created by creating a polygon from a list of coordinates or MKMapPoint
. The polygon appearance can then be modified with style, stroke, and tint modifiers.
import SwiftUI
import MapKit
struct AreaOnMapView: View {
let square: [CLLocationCoordinate2D] = [
CLLocationCoordinate2D(latitude: 40.836542, longitude: 14.307110),
CLLocationCoordinate2D(latitude: 40.836736, longitude: 14.307022),
CLLocationCoordinate2D(latitude: 40.836418, longitude: 14.305917),
CLLocationCoordinate2D(latitude: 40.836218, longitude: 14.305998)
]
var body: some View {
Map {
MapPolygon(coordinates: square)
.foregroundStyle(.pink.opacity(0.7))
}
}
}
In the code above we are drawing a polygon based on 4 coordinates, resulting on a rectangle drawn on the map.
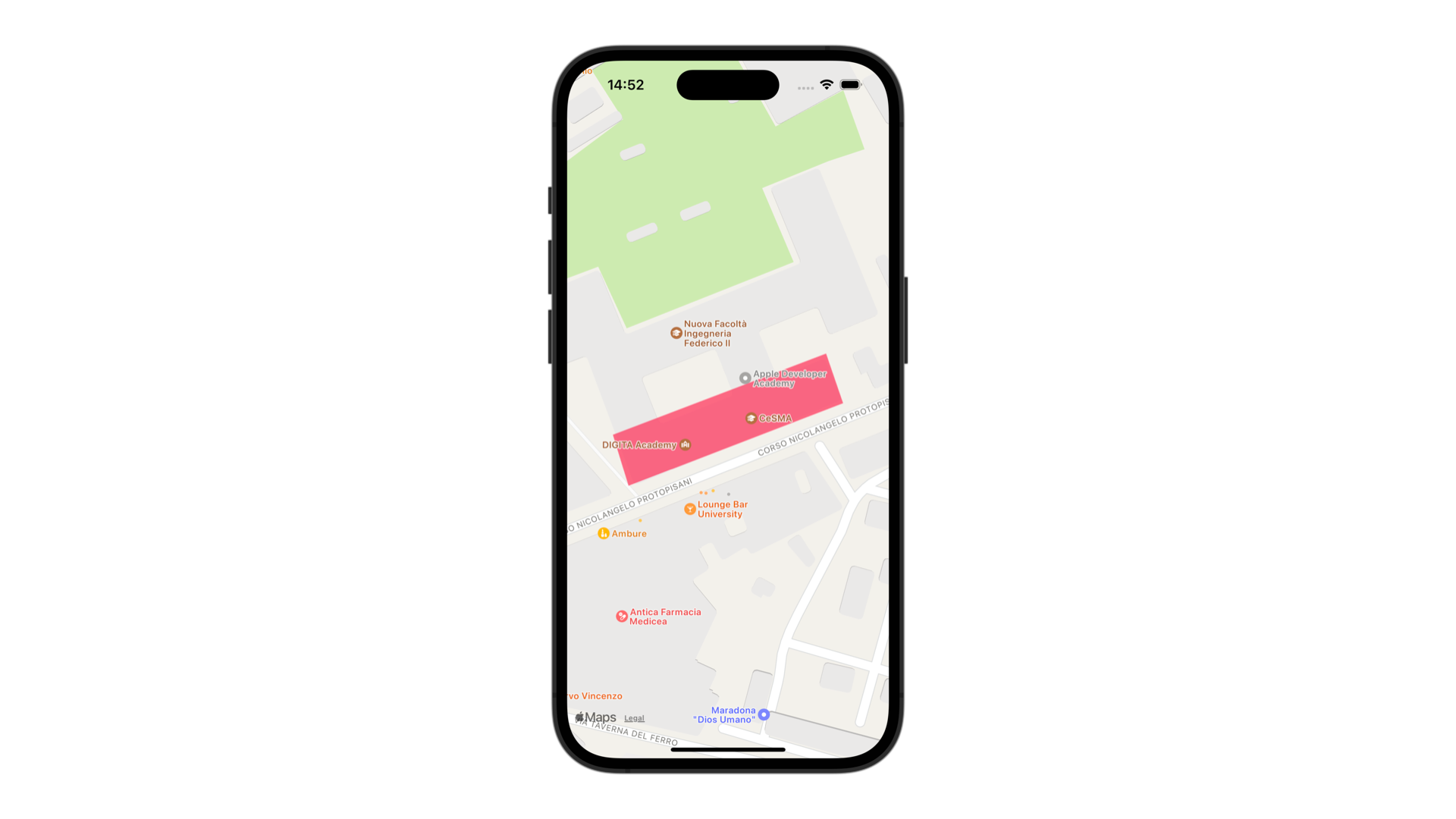
You can achieve the same result using MKMapPoint
objects on an array.
struct AreaOnMapView: View {
let points: [MKMapPoint] = [
MKMapPoint(CLLocationCoordinate2D(
latitude: 40.836542,
longitude: 14.307110
)),
MKMapPoint(CLLocationCoordinate2D(
latitude: 40.836736,
longitude: 14.307022
)),
MKMapPoint(CLLocationCoordinate2D(
latitude: 40.836418,
longitude: 14.305917
)),
MKMapPoint(CLLocationCoordinate2D(
latitude: 40.836218,
longitude: 14.305998
))
]
var body: some View {
Map {
MapPolygon(points: points)
.foregroundStyle(.pink.opacity(0.7))
}
}
}
As mentioned before, you can use modifiers to change how the polygon looks. To know more about which modifiers are available, check the documentation page for MapPolygon
.
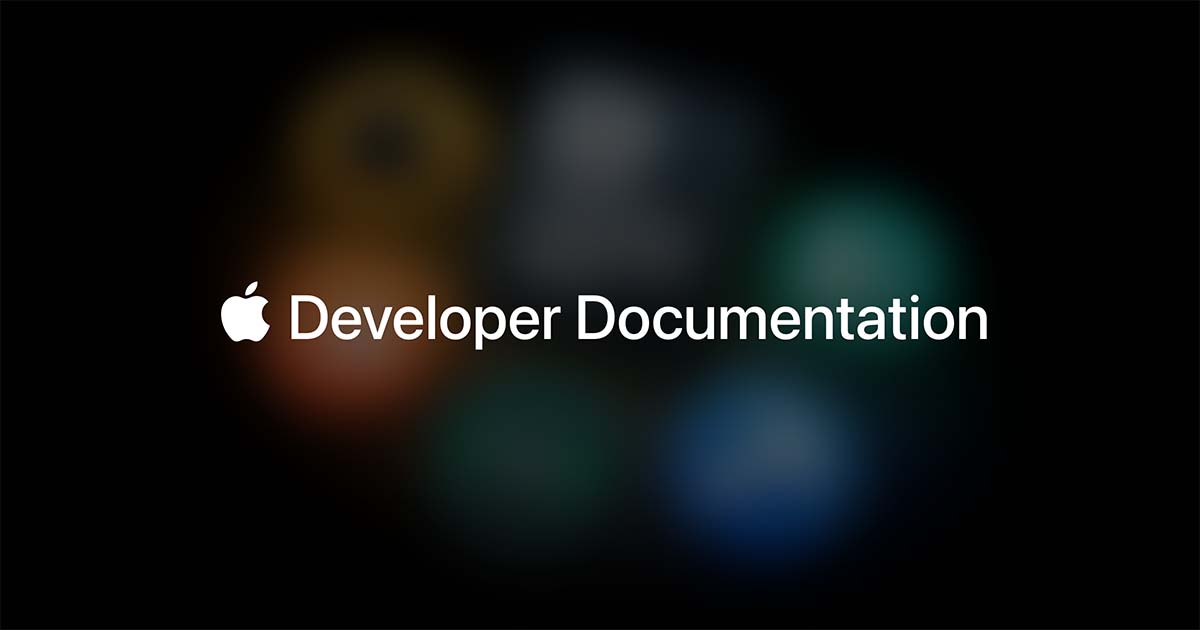