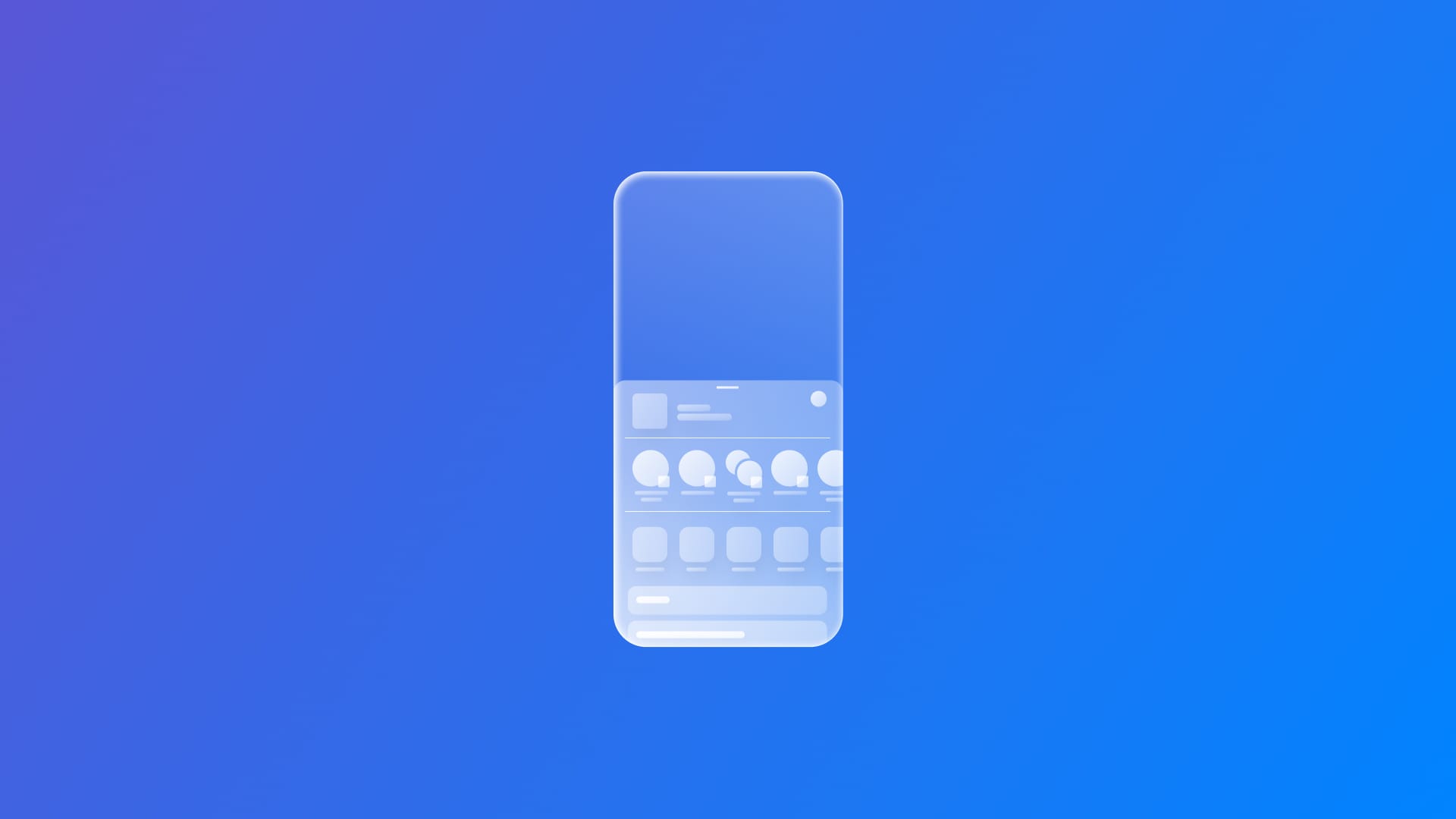
Using the share sheet to share content in a SwiftUI app
Enable sharing content from your app using the system share sheet within a SwiftUI app
Sharing data among different applications is an everyday use case. You might share contact information, an image from your camera app, or a simple text as a new message.
In SwiftUI, to share various types of data from an application with other applications, use the ShareLink
view. At Apple’s Human Interface Guidelines, this view controls a sharing presentation called Activity Views.
ShareLink(item: URL(string: "https://www.createwithswift.com")!)
<– Simple_ShareLink.mp4: Showing the ShareLink component without a Label -->
Other ways to initialize a share link component include defining a custom label or providing a customized title and description for the item to be shared.
// With customize link title
ShareLink("Share Website", item: URL(string: "https://www.createwithswift.com")!)
// With a customized sharing subject and message
ShareLink(
item: URL(string: "https://www.createwithswift.com")!,
subject: Text("Share website"),
message: Text("Share Create with Swift with your friends")
)
Section("With a Preview") {
ShareLink(
item: URL(string: "https://www.createwithswift.com/napoli-meetup")!,
preview: SharePreview("Join the meetup!", icon: Image("meetup-icon"))
)
}
We can flexibly control the link’s appearance using the Label
to define the displayed text and a custom icon.
ShareLink(item: URL(string: "https://www.createwithswift.com")!) {
Label("Share website", systemImage: "square.and.arrow.up.fill")
}
Different transferable types
The content shared through a ShareLink
view must conform to the Transferable
protocol. Some types already conform to it, such as URL
, but you can also make custom types transferable by adopting the protocol yourself.
struct SocialMediaPost: Transferable {
static var transferRepresentation: some TransferRepresentation {
ProxyRepresentation(exporting: \.image)
}
public var image: Image
public var title: String
public var description: String
}
struct SocialMediaPostView: View {
let post: SocialMediaPost
var body: some View {
VStack(alignment: .leading, spacing: 10) {
// Image of the post
post.image
.resizable()
.aspectRatio(contentMode: .fill)
VStack(alignment: .leading, spacing: 16) {
// Actions on the post
HStack {
Button("Like", systemImage: "heart.fill") {
// Like action here
}
.labelStyle(.iconOnly)
Spacer()
ShareLink(
item: post,
preview: SharePreview(post.title, image: post.image)
)
.labelStyle(.iconOnly)
}
Text(post.description)
}
.padding()
}
}
}
For your custom types, use ProxyRepresentation
to specify which portion of the information will be shared when sharing it via the share sheet. To define how the information will be previewed, you can use SharePreview
and specify a title, an image and an icon to be presented on the system’s default sharing sheet.
By understanding how to use ShareLink
and the Transferable
protocol, you will be able to integrate your application with the system sharing functionalities and extend what your user can accomplish with it.